1.创建一个employee类,该类中有姓名、城市、邮政编码等数据成员。成员函数setValue设定对象数据,display函数输出对象数据。 具体要求如下: (1)数据成员设定成private (2)成员函数设定成public (3)建立指针对象,利用指针对象对成员进行引用 (4)将类声明和成员函数的定义分开,放到.h和.cpp中
时间: 2024-02-12 12:09:36 浏览: 96
好的,下面是对应的头文件和源文件:
employee.h:
```c++
#ifndef EMPLOYEE_H
#define EMPLOYEE_H
#include <string>
using namespace std;
class Employee {
private:
string name;
string city;
string postal_code;
public:
void setValue(string name, string city, string postal_code);
void display();
};
#endif
```
employee.cpp:
```c++
#include <iostream>
#include "employee.h"
using namespace std;
void Employee::setValue(string name, string city, string postal_code) {
this->name = name;
this->city = city;
this->postal_code = postal_code;
}
void Employee::display() {
cout << "Name: " << name << endl;
cout << "City: " << city << endl;
cout << "Postal code: " << postal_code << endl;
}
```
然后在主函数中使用指针对象对成员进行引用:
```c++
#include "employee.h"
using namespace std;
int main() {
Employee* emp = new Employee();
emp->setValue("Tom", "New York", "12345");
emp->display();
delete emp;
return 0;
}
```
运行结果如下:
```
Name: Tom
City: New York
Postal code: 12345
```
阅读全文
相关推荐
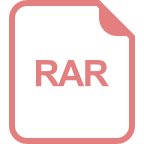
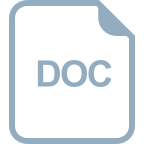
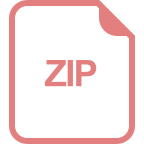











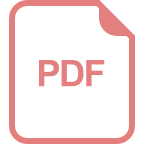
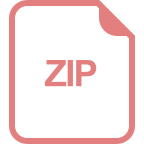
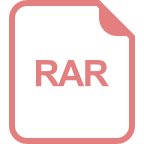
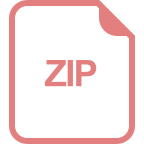
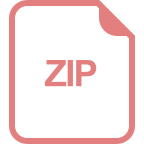