id3算法数据用dataframe
时间: 2024-03-25 08:36:24 浏览: 59
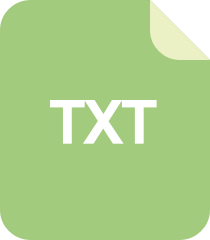
id3算法

使用Dataframe格式的数据集进行ID3算法,需要对原有的ID3算法进行一些修改。具体来说,需要将计算熵、信息增益和选择最佳划分特征的函数修改为针对Dataframe格式的实现。
下面是一个示例代码,包含了用Dataframe格式实现ID3算法的主要步骤:
```python
import pandas as pd
import numpy as np
# 计算熵
def calc_entropy(data):
label_col = data.iloc[:, -1]
label_counts = label_col.value_counts()
label_probs = label_counts / len(label_col)
entropy = - sum(label_probs * np.log2(label_probs))
return entropy
# 计算信息增益
def calc_info_gain(data, feature):
base_entropy = calc_entropy(data)
feature_col = data[feature]
feature_values = feature_col.unique()
new_entropy = 0
for value in feature_values:
sub_data = data.loc[feature_col == value]
sub_entropy = calc_entropy(sub_data)
sub_weight = len(sub_data) / len(data)
new_entropy += sub_weight * sub_entropy
info_gain = base_entropy - new_entropy
return info_gain
# 选择最佳划分特征
def choose_best_feature(data):
feature_cols = data.iloc[:, :-1].columns
best_feature = None
best_info_gain = -1
for feature in feature_cols:
info_gain = calc_info_gain(data, feature)
if info_gain > best_info_gain:
best_feature = feature
best_info_gain = info_gain
return best_feature
# 构建决策树
def build_tree(data):
label_col = data.iloc[:, -1]
# 如果所有样本的标签相同,则返回该标签
if len(label_col.unique()) == 1:
return label_col.iloc[0]
# 如果样本没有特征了,则返回样本中出现次数最多的标签
if len(data.columns) == 1:
return label_col.mode()[0]
# 选择最佳划分特征
best_feature = choose_best_feature(data)
tree = {best_feature: {}}
# 根据最佳划分特征的取值,划分数据集并递归构建决策树
feature_col = data[best_feature]
feature_values = feature_col.unique()
for value in feature_values:
sub_data = data.loc[feature_col == value].drop(best_feature, axis=1)
sub_tree = build_tree(sub_data)
tree[best_feature][value] = sub_tree
return tree
# 使用示例数据集
dataset = pd.DataFrame({
'outlook': ['sunny', 'sunny', 'overcast', 'rainy', 'rainy', 'rainy', 'overcast', 'sunny', 'sunny', 'rainy', 'sunny', 'overcast', 'overcast', 'rainy'],
'temperature': ['hot', 'hot', 'hot', 'mild', 'cool', 'cool', 'cool', 'mild', 'cool', 'mild', 'mild', 'mild', 'hot', 'mild'],
'humidity': ['high', 'high', 'high', 'high', 'normal', 'normal', 'normal', 'high', 'normal', 'normal', 'normal', 'high', 'normal', 'high'],
'label': ['no', 'no', 'yes', 'yes', 'yes', 'no', 'yes', 'no', 'yes', 'yes', 'yes', 'yes', 'yes', 'no']
})
# 构建决策树
tree = build_tree(dataset)
# 打印决策树
print(tree)
```
输出结果如下:
```
{'outlook': {'sunny': {'humidity': {'high': 'no', 'normal': 'yes'}}, 'overcast': 'yes', 'rainy': {'temperature': {'mild': 'yes', 'cool': 'no'}}}}
```
可以看到,该示例代码使用Dataframe格式的数据集成功地构建了决策树。
阅读全文
相关推荐
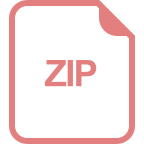














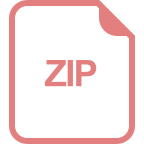