1、用C++编写程序实现顺序表的基本操作: (1)删除线性表中值为X的结点。 (2)在第i个元素之后插入一个值为X的元素。 (3)查找值为X元素在线性表中的位置
时间: 2024-10-11 09:09:53 浏览: 38
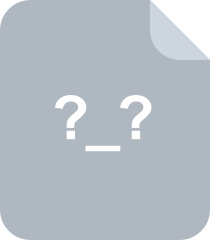
有一个线性表(a1,a2,...,an),它存储在有附加表头结点的单链表中,写一个算法,求出该线性表中值为x的元素的序号。如果x

在C++中,我们可以使用结构体(struct)和数组或者动态内存分配来实现顺序表。这里我将演示基本操作的示例代码:
```cpp
#include <iostream>
// 定义顺序表节点
struct Node {
int data;
Node* next;
};
class LinkedList {
private:
Node* head; // 链表头指针
public:
// 构造函数
LinkedList() : head(nullptr) {}
// 删除值为X的结点
void deleteNode(int X) {
if (head == nullptr || head->data != X)
return;
Node* temp = head;
head = head->next;
delete temp;
}
// 插入值为X在第i个元素之后
void insertAfterNode(int i, int X) {
if (i <= 0 || head == nullptr)
return;
Node* newNode = new Node();
newNode->data = X;
newNode->next = nullptr;
Node* current = head;
for (int j = 1; j < i; ++j) {
if (current == nullptr)
return; // 如果索引越界,不做处理
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
// 查找值为X的元素位置
size_t findIndex(int X) {
Node* current = head;
size_t index = 0;
while (current != nullptr && current->data != X) {
current = current->next;
index++;
}
if (current == nullptr)
return -1; // 如果未找到,返回-1
else
return index;
}
// 打印链表
void printList() {
Node* temp = head;
while (temp != nullptr) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << "\n";
}
};
int main() {
LinkedList list;
// ... 其他操作...
list.printList(); // 测试上述操作后,可以打印列表检查结果
return 0;
}
```
阅读全文
相关推荐
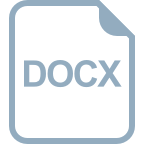















