string line; string rgb_file, depth_file; double time_rgb, time_depth; while (getline(fin, line)) { istringstream iss(line); iss >> time_rgb >> rgb_file >> time_depth >> depth_file; // 处理读取到的数据 cout << "RGB文件:" << rgb_file << endl; cout << "深度文件:" << depth_file << endl; cout << "RGB时间戳:" << time_rgb << endl; cout << "深度时间戳:" << time_depth << endl; // TODO: 将RGB和深度图像数据进行匹配等操作 } // 关闭文件流 fin.close();这段代码把double改成long可以吗?
时间: 2024-03-29 17:36:08 浏览: 12
可以,将double改成long是可以的,只需要将代码中的double改为long即可。但是需要注意的是,将时间戳从double类型改为long类型可能会导致精度丢失,因为long类型只能表示整数,而double类型可以表示小数。因此,如果时间戳的精度对你的应用非常重要,最好还是使用double类型。
相关问题
fin >> time_rgb >> rgb_file >> time_depth >> depth_file;ifstream fin(associate_file);c++中如何利用associate.txt文件读取数据
您可以使用C++中的文件流(fstream)来读取文件数据。具体步骤如下:
1. 打开associate.txt文件,创建输入文件流对象,将文件名作为参数传入构造函数中:
```
ifstream fin(associate_file);
```
2. 在循环中读取每一行数据。可以使用while循环和getline()函数来实现。同时,为了方便起见,我们可以定义一些变量来存储每一行数据中的信息:
```
string rgb_file, depth_file;
double time_rgb, time_depth;
while (getline(fin, line)) {
istringstream iss(line);
iss >> time_rgb >> rgb_file >> time_depth >> depth_file;
// TODO: 处理读取到的数据
}
```
3. 在读取到每一行数据后,您可以对数据进行处理,例如将RGB和深度图像数据进行匹配等。
完整的代码如下所示:
```
#include <iostream>
#include <fstream>
#include <sstream>
using namespace std;
int main() {
// 打开associate.txt文件,创建输入文件流对象
string associate_file = "associate.txt";
ifstream fin(associate_file);
if (!fin) {
cerr << "无法打开文件" << associate_file << endl;
return 1;
}
// 逐行读取数据
string line;
string rgb_file, depth_file;
double time_rgb, time_depth;
while (getline(fin, line)) {
istringstream iss(line);
iss >> time_rgb >> rgb_file >> time_depth >> depth_file;
// 处理读取到的数据
cout << "RGB文件:" << rgb_file << endl;
cout << "深度文件:" << depth_file << endl;
cout << "RGB时间戳:" << time_rgb << endl;
cout << "深度时间戳:" << time_depth << endl;
// TODO: 将RGB和深度图像数据进行匹配等操作
}
// 关闭文件流
fin.close();
return 0;
}
```
在这个例子中,我们打开了associate.txt文件,并逐行读取文件中的数据。将每一行数据中的RGB文件名、深度文件名、RGB时间戳和深度时间戳存储在变量中,并对它们进行了简单的处理和输出。最后,关闭文件流。
ifstream fin(associate_file); if (!fin) { cerr << "I cann't find associate.txt!" << endl; return 1; } string rgb_file, depth_file, time_rgb, time_depth; list< cv::Point2f > keypoints; // 因为要删除跟踪失败的点,使用list cv::Mat color, depth, last_color; for (int index = 0; index < 100; index++) { fin >> time_rgb >> rgb_file >> time_depth >> depth_file; color = cv::imread(path_to_dataset + "/" + rgb_file); depth = cv::imread(path_to_dataset + "/" + depth_file, -1);这段代码是不是没有对fiin读取一行的操作
是的,这段代码没有显式地调用 `fin` 的 `getline()` 或 `>>` 操作符来读取一整行,而是直接用 `fin >> ... >> ... >> ...` 的方式逐个读取 `fin` 中的每个单词。这种方式只能保证在单词之间正确分割字符串,但无法保证每行都能完整读取。如果一行中的单词数目不符合预期,或者某个单词中包含空格、制表符等空白字符,就会导致读取出错。因此,建议使用 `getline()` 函数来逐行读取文本文件。例如,可以改为以下代码:
```cpp
ifstream fin(associate_file);
if (!fin) {
cerr << "I can't find associate.txt!" << endl;
return 1;
}
string line;
while (getline(fin, line)) {
istringstream iss(line);
string time_rgb, rgb_file, time_depth, depth_file;
if (!(iss >> time_rgb >> rgb_file >> time_depth >> depth_file)) {
cerr << "Error reading line: " << line << endl;
continue;
}
cv::Mat color = cv::imread(path_to_dataset + "/" + rgb_file);
cv::Mat depth = cv::imread(path_to_dataset + "/" + depth_file, -1);
// ...
}
```
这样可以保证每次读取一整行,并且使用 `istringstream` 来解析每行中的单词。如果读取失败,可以输出错误信息并跳过当前行。
相关推荐
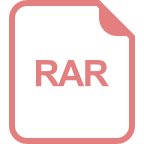
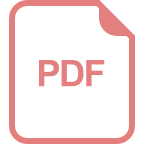












