写一个完整的python车牌识别系统
时间: 2023-03-14 15:33:18 浏览: 77
我们可以使用Python开发一个完整的车牌识别系统,这个系统可以从图像中识别出车牌。首先,我们需要使用OpenCV库或其他工具从图像中提取出车牌,然后使用Python TensorFlow库对车牌进行分类,最后使用Python Pillow库来识别车牌上的文字并获取车牌号码。
相关问题
用python写一个车牌识别系统
要实现一个车牌识别系统,需要先准备好以下工具和库:
- Python 3.x
- OpenCV
- NumPy
- Pytesseract
- Tesseract OCR
以下是一个基本的车牌识别系统的代码示例:
```python
import cv2
import numpy as np
import pytesseract
# 读取图片
img = cv2.imread('car_plate.jpg')
# 图像预处理
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
gray = cv2.GaussianBlur(gray, (5, 5), 0)
edged = cv2.Canny(gray, 50, 200)
contours, hierarchy = cv2.findContours(edged.copy(), cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
contours = sorted(contours, key=cv2.contourArea, reverse=True)[:10]
screenCnt = None
# 找到车牌轮廓
for c in contours:
peri = cv2.arcLength(c, True)
approx = cv2.approxPolyDP(c, 0.02 * peri, True)
if len(approx) == 4:
screenCnt = approx
break
# 将车牌区域抠出来并识别
if screenCnt is not None:
mask = np.zeros(gray.shape, np.uint8)
cv2.drawContours(mask, [screenCnt], 0, 255, -1)
newImg = cv2.bitwise_and(img, img, mask=mask)
gray_new = cv2.cvtColor(newImg, cv2.COLOR_BGR2GRAY)
thresh = cv2.threshold(gray_new, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)[1]
text = pytesseract.image_to_string(thresh, lang='eng', config='--psm 11')
print('车牌号码为:', text)
```
这个代码片段中,我们首先读取输入的图像,然后对图像进行预处理,包括灰度化、高斯模糊、边缘检测和轮廓检测等操作。接着,我们找到车牌的轮廓,将车牌区域抠出来,并使用 pytesseract 库对车牌区域进行 OCR 识别。最后输出识别结果。
如何用python写一个车牌识别系统
很高兴回答你的问题。您可以通过以下步骤使用Python构建车牌识别系统:
1.使用Python和OpenCV库读取和处理图像
2.在处理的图像中使用图像处理技术和算法以提取车牌号码
3.使用机器学习算法训练一个字符识别模型,可以将车牌号码的字符识别出来
4.将字符识别出来的结果进行整理、清洗和验证,以得到最终的车牌号码
您可以使用开源库和模型,例如OpenALPR等,以便更快速地实现该系统。希望这可以帮助您开始构建您的车牌识别系统!
相关推荐
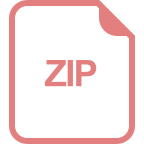
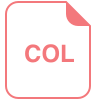
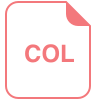
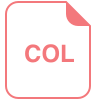
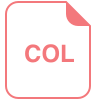
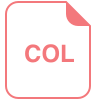






