c#访问共享文件夹地址
时间: 2023-08-02 11:28:29 浏览: 245
你可以使用 C# 中的 `System.IO` 命名空间下的 `Directory` 类和 `File` 类来访问共享文件夹地址。下面是一个简单的示例代码:
```csharp
using System.IO;
// 访问共享文件夹
string path = @"\\server\share\folder";
// 判断文件夹是否存在
if (Directory.Exists(path))
{
Console.WriteLine("共享文件夹已存在!");
// 获取共享文件夹下的文件列表
string[] files = Directory.GetFiles(path);
foreach (string file in files)
{
Console.WriteLine(file);
}
}
else
{
Console.WriteLine("共享文件夹不存在!");
}
```
在访问共享文件夹时,需要确保你的程序有访问该共享文件夹的权限。
相关问题
c# 访问共享文件夹
在 C# 中访问共享文件夹,可以使用 System.IO 命名空间下的类来实现。以下是一个简单的示例代码:
```csharp
using System.IO;
// 访问共享文件夹
string path = @"\\server\share\file.txt";
string username = "username"; // 共享文件夹的用户名
string password = "password"; // 共享文件夹的密码
// 创建网络凭据对象
var networkCredential = new System.Net.NetworkCredential(username, password);
// 设置访问共享文件夹的权限
var permission = new FileIOPermission(FileIOPermissionAccess.Read, path);
permission.AddPathList(FileIOPermissionAccess.Read, path);
permission.Demand();
// 访问共享文件夹
using (new System.Net.NetworkCredential("", password, username))
{
// 读取共享文件夹中的文件内容
string content = File.ReadAllText(path);
Console.WriteLine(content);
}
```
其中,`path` 表示共享文件夹的路径,`username` 和 `password` 分别表示共享文件夹的用户名和密码。首先需要创建一个网络凭据对象,并设置访问共享文件夹的权限。然后使用 `File.ReadAllText` 方法读取共享文件夹中的文件内容。注意,在访问共享文件夹时需要提供正确的用户名和密码。
c#访问共享文件夹文件
在 C# 中访问共享文件夹文件,你可以使用 System.IO 命名空间下的 DirectoryInfo 和 FileInfo 类。首先,你需要指定共享文件夹所在的计算机名称和共享名称,例如:
```
string path = @"\\COMPUTER_NAME\SHARED_FOLDER\file.txt";
```
然后,你可以使用 DirectoryInfo 和 FileInfo 类中的方法访问该文件,例如:
```
// 获取共享文件夹中的所有文件
DirectoryInfo directory = new DirectoryInfo(@"\\COMPUTER_NAME\SHARED_FOLDER");
FileInfo[] files = directory.GetFiles();
// 访问特定文件
FileInfo file = new FileInfo(@"\\COMPUTER_NAME\SHARED_FOLDER\file.txt");
string content = File.ReadAllText(file.FullName);
```
需要注意的是,你需要确保你的程序具有访问共享文件夹的权限,并且共享文件夹的访问权限设置正确。
阅读全文
相关推荐
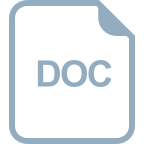
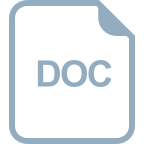
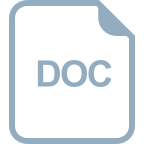
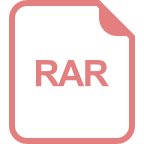


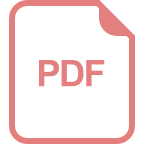
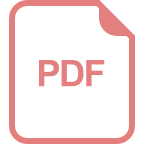
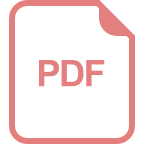
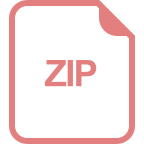






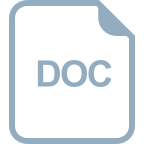