编写一个Java程序,要求如下:分别使用节点流:FileInputStream、FileOutputStream和缓冲流:BufferedInputStream、BufferedOutputStream实现文本文件/图片/视频文件的复制。并比较二者在数据复制方面的效率。 【提示】 (1)使用单元测试完成编程,如下: @Test public void testFileCopy(){ // 代码部分 ……} (2)被复制的文件名为:dog.jpg ,复制后的文件名:dog_copy.jpg (3)在进行文件复制操作中,遇到的异常都抛出(使用thorows)。
时间: 2024-02-18 21:05:00 浏览: 122
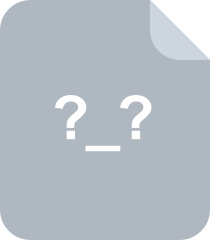
用流FileInputStream FileOutputStream 实现文件复制

以下是一个实现上述要求的Java程序,包括了节点流和缓冲流两种方式的复制实现,并使用JUnit进行单元测试:
```
import org.junit.Test;
import java.io.*;
public class FileCopyTest {
// 使用节点流实现文件复制
public void copyByNodeStream(File source, File target) throws IOException {
try (FileInputStream fis = new FileInputStream(source);
FileOutputStream fos = new FileOutputStream(target)) {
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
}
}
// 使用缓冲流实现文件复制
public void copyByBufferedStream(File source, File target) throws IOException {
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream(source));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(target))) {
byte[] buffer = new byte[1024];
int len;
while ((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
}
}
@Test
public void testFileCopy() throws IOException {
File source = new File("dog.jpg");
File target1 = new File("dog_copy_node.jpg");
File target2 = new File("dog_copy_buffered.jpg");
// 使用节点流复制文件并计时
long startTime1 = System.currentTimeMillis();
copyByNodeStream(source, target1);
long endTime1 = System.currentTimeMillis();
System.out.println("节点流复制文件用时:" + (endTime1 - startTime1) + "毫秒");
// 使用缓冲流复制文件并计时
long startTime2 = System.currentTimeMillis();
copyByBufferedStream(source, target2);
long endTime2 = System.currentTimeMillis();
System.out.println("缓冲流复制文件用时:" + (endTime2 - startTime2) + "毫秒");
}
}
```
在上述程序中,我们定义了两个方法`copyByNodeStream()`和`copyByBufferedStream()`,分别使用节点流和缓冲流实现文件复制。然后在`testFileCopy()`方法中,我们对这两种方式进行测试,并输出复制所需时间,以便比较二者效率。
注意,在程序中我们使用了Java 7以后引入的try-with-resources语法,可以自动关闭资源,无需手动调用close()方法。同时,我们也使用了JUnit进行单元测试,以保证程序的正确性。
阅读全文
相关推荐
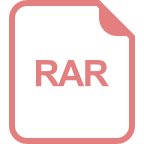
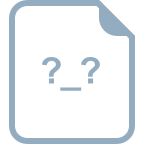

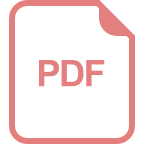
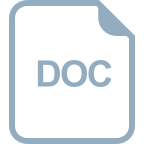
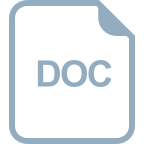
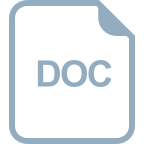
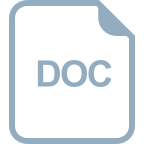
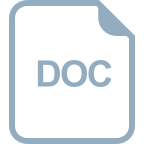
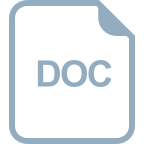
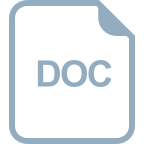
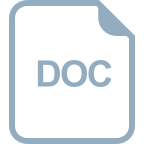
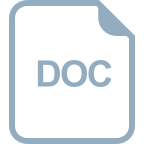
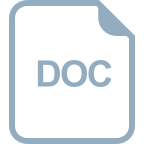
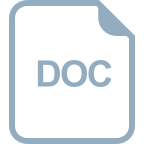
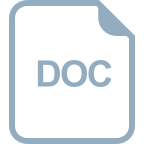
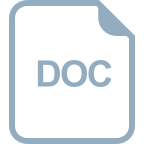
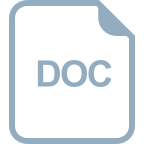