w5500 stm32开发hal库
时间: 2023-04-07 21:00:55 浏览: 166
我可以回答这个问题。w5500是一款基于TCP/IP协议的以太网控制器,而STM32是一款微控制器,HAL库是STM32的一个软件库,用于简化开发者的编程工作。因此,w5500 stm32开发hal库是指在STM32上使用HAL库来开发w5500以太网控制器。
相关问题
stm32f103 hal库驱动W5500代码
以下是使用STM32CubeMX和HAL库驱动W5500的代码示例,使用SPI通信:
```
#include "main.h"
#include "spi.h"
#include "gpio.h"
#include "w5500.h"
#include "socket.h"
#define WIZ_RESET_PORT GPIOA
#define WIZ_RESET_PIN GPIO_PIN_1
void WIZ_CS_Select(void)
{
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_12, GPIO_PIN_RESET);
}
void WIZ_CS_Deselect(void)
{
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_12, GPIO_PIN_SET);
}
void WIZ_SPI_Transmit(uint8_t *buf, uint16_t len)
{
HAL_SPI_Transmit(&hspi1, buf, len, 1000);
}
void WIZ_SPI_Receive(uint8_t *buf, uint16_t len)
{
HAL_SPI_Receive(&hspi1, buf, len, 1000);
}
void WIZ_Delay_ms(uint32_t ms)
{
HAL_Delay(ms);
}
int main(void)
{
HAL_Init();
MX_GPIO_Init();
MX_SPI1_Init();
WIZ_RESET_PORT->BSRR = WIZ_RESET_PIN; // Deassert reset
HAL_Delay(10);
WIZ_RESET_PORT->BRR = WIZ_RESET_PIN; // Assert reset
HAL_Delay(10);
WIZ_RESET_PORT->BSRR = WIZ_RESET_PIN; // Deassert reset
HAL_Delay(1000); // Wait for W5500 to initialize
uint8_t mac_addr[6] = {0x00, 0x08, 0xDC, 0x11, 0x22, 0x33};
uint8_t ip_addr[4] = {192, 168, 0, 10};
uint8_t gateway_addr[4] = {192, 168, 0, 1};
uint8_t subnet_mask[4] = {255, 255, 255, 0};
WIZ_Init(mac_addr, ip_addr, gateway_addr, subnet_mask);
while (1)
{
uint8_t socket_num = 0;
uint16_t port = 80;
uint8_t server_ip_addr[4] = {192, 168, 0, 2};
uint16_t server_port = 8080;
// Open socket
uint8_t socket_status = 0;
socket_num = socket(0, Sn_MR_TCP, port, 0);
if (socket_num != 0xFF)
{
socket_status = getSn_SR(socket_num);
if (socket_status == SOCK_INIT)
{
// Connect to server
connect(socket_num, server_ip_addr, server_port);
socket_status = getSn_SR(socket_num);
if (socket_status == SOCK_ESTABLISHED)
{
// Send data to server
uint8_t tx_buf[] = "Hello, server!";
send(socket_num, tx_buf, sizeof(tx_buf));
socket_status = getSn_SR(socket_num);
if (socket_status == SOCK_ESTABLISHED)
{
// Receive data from server
uint8_t rx_buf[1024] = {0};
uint16_t rx_len = recv(socket_num, rx_buf, sizeof(rx_buf));
if (rx_len > 0)
{
// Process received data
}
}
}
// Close socket
close(socket_num);
}
}
HAL_Delay(1000);
}
}
```
在此示例中,`WIZ_Init`函数用于初始化W5500,`socket`、`connect`、`send`和`recv`函数用于与服务器进行TCP通信。注意,在使用W5500之前需要正确配置引脚、SPI和时钟。
stm32f103 hal库驱动W5500详细代码
以下是基于STM32CubeIDE和HAL库的W5500驱动代码:
首先,在CubeMX中配置GPIO和SPI,然后生成代码。
然后,在main.c文件中初始化W5500并设置其寄存器。
```c
#include "main.h"
#include "spi.h"
#include "gpio.h"
#include "w5500.h"
W5500_Dev w5500_dev;
int main(void)
{
HAL_Init();
MX_GPIO_Init();
MX_SPI1_Init();
W5500_Init(&w5500_dev, &hspi1, GPIOA, GPIO_PIN_4);
W5500_SetMACAddress(&w5500_dev, (uint8_t *)"AA:BB:CC:DD:EE:FF");
W5500_SetIPAddress(&w5500_dev, 192, 168, 1, 100);
W5500_SetGatewayAddress(&w5500_dev, 192, 168, 1, 1);
W5500_SetSubnetMask(&w5500_dev, 255, 255, 255, 0);
while (1)
{
// loop
}
}
```
在w5500.h头文件中定义了驱动的API函数。
```c
#ifndef __W5500_H
#define __W5500_H
#ifdef __cplusplus
extern "C" {
#endif
#include "stm32f1xx_hal.h"
#define W5500_REGISTER_BLOCK_SIZE 0x400
#define W5500_REGISTER_BLOCK_SIZE_SHIFT 10
typedef struct
{
SPI_HandleTypeDef *spi;
GPIO_TypeDef *cs_port;
uint16_t cs_pin;
} W5500_Dev;
void W5500_Init(W5500_Dev *dev, SPI_HandleTypeDef *spi, GPIO_TypeDef *cs_port, uint16_t cs_pin);
void W5500_SetMACAddress(W5500_Dev *dev, uint8_t *mac);
void W5500_SetIPAddress(W5500_Dev *dev, uint8_t ip1, uint8_t ip2, uint8_t ip3, uint8_t ip4);
void W5500_SetGatewayAddress(W5500_Dev *dev, uint8_t ip1, uint8_t ip2, uint8_t ip3, uint8_t ip4);
void W5500_SetSubnetMask(W5500_Dev *dev, uint8_t ip1, uint8_t ip2, uint8_t ip3, uint8_t ip4);
#ifdef __cplusplus
}
#endif
#endif /* __W5500_H */
```
在w5500.c文件中实现了这些API函数。
```c
#include "w5500.h"
#define W5500_SPI_TIMEOUT 1000
#define W5500_REGISTER_MODE 0x0000
#define W5500_REGISTER_GATEWAY_ADDRESS 0x0001
#define W5500_REGISTER_SUBNET_MASK 0x0005
#define W5500_REGISTER_MAC_ADDRESS 0x0009
#define W5500_REGISTER_IP_ADDRESS 0x000F
#define W5500_MODE_VALUE 0x01
void W5500_Init(W5500_Dev *dev, SPI_HandleTypeDef *spi, GPIO_TypeDef *cs_port, uint16_t cs_pin)
{
dev->spi = spi;
dev->cs_port = cs_port;
dev->cs_pin = cs_pin;
HAL_GPIO_WritePin(dev->cs_port, dev->cs_pin, GPIO_PIN_SET);
uint8_t tx_data[3] = {0x00, 0x00, W5500_MODE_VALUE};
uint8_t rx_data[3] = {0};
HAL_GPIO_WritePin(dev->cs_port, dev->cs_pin, GPIO_PIN_RESET);
HAL_SPI_TransmitReceive(dev->spi, tx_data, rx_data, sizeof(tx_data), W5500_SPI_TIMEOUT);
HAL_GPIO_WritePin(dev->cs_port, dev->cs_pin, GPIO_PIN_SET);
}
void W5500_SetMACAddress(W5500_Dev *dev, uint8_t *mac)
{
uint8_t tx_data[7] = {0x00, 0x09, mac[0], mac[1], mac[2], mac[3], mac[4]};
HAL_GPIO_WritePin(dev->cs_port, dev->cs_pin, GPIO_PIN_RESET);
HAL_SPI_Transmit(dev->spi, tx_data, sizeof(tx_data), W5500_SPI_TIMEOUT);
HAL_GPIO_WritePin(dev->cs_port, dev->cs_pin, GPIO_PIN_SET);
}
void W5500_SetIPAddress(W5500_Dev *dev, uint8_t ip1, uint8_t ip2, uint8_t ip3, uint8_t ip4)
{
uint8_t tx_data[5] = {0x00, 0x0F, ip1, ip2, ip3, ip4};
HAL_GPIO_WritePin(dev->cs_port, dev->cs_pin, GPIO_PIN_RESET);
HAL_SPI_Transmit(dev->spi, tx_data, sizeof(tx_data), W5500_SPI_TIMEOUT);
HAL_GPIO_WritePin(dev->cs_port, dev->cs_pin, GPIO_PIN_SET);
}
void W5500_SetGatewayAddress(W5500_Dev *dev, uint8_t ip1, uint8_t ip2, uint8_t ip3, uint8_t ip4)
{
uint8_t tx_data[5] = {0x00, 0x01, ip1, ip2, ip3, ip4};
HAL_GPIO_WritePin(dev->cs_port, dev->cs_pin, GPIO_PIN_RESET);
HAL_SPI_Transmit(dev->spi, tx_data, sizeof(tx_data), W5500_SPI_TIMEOUT);
HAL_GPIO_WritePin(dev->cs_port, dev->cs_pin, GPIO_PIN_SET);
}
void W5500_SetSubnetMask(W5500_Dev *dev, uint8_t ip1, uint8_t ip2, uint8_t ip3, uint8_t ip4)
{
uint8_t tx_data[5] = {0x00, 0x05, ip1, ip2, ip3, ip4};
HAL_GPIO_WritePin(dev->cs_port, dev->cs_pin, GPIO_PIN_RESET);
HAL_SPI_Transmit(dev->spi, tx_data, sizeof(tx_data), W5500_SPI_TIMEOUT);
HAL_GPIO_WritePin(dev->cs_port, dev->cs_pin, GPIO_PIN_SET);
}
```
这些函数中,主要的操作是通过SPI总线向W5500写入寄存器的值。每个寄存器都有一个地址,可以在W5500的数据手册中找到。
注意,这里只给出了一些基本的初始化函数,还可以写更多的函数来配置其他寄存器。同时,还需要编写其他函数来读取和写入数据包以进行网络通信。
阅读全文
相关推荐
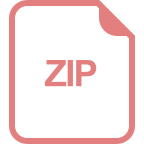
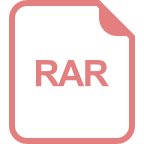
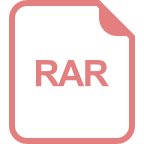
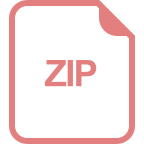
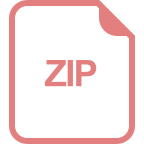
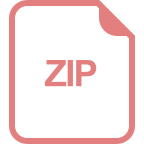
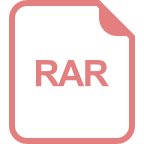



