,编程实现基于Adaboost的目标检测算法
时间: 2023-12-14 19:17:49 浏览: 35
好的,下面我将为您介绍基于Adaboost的目标检测算法的编程实现步骤,希望对您有所帮助。
1. 数据集准备:选择一个合适的目标检测数据集,并进行数据预处理,包括图像的缩放、裁剪、灰度化等操作。同时需要为每个图像标注目标位置的ground truth。
2. 特征提取:选择一种适合于目标检测的特征描述子,常用的有HOG、LBP、Haar-like等。对于每个图像,提取目标区域的特征描述子,并将其转化为分类器所需的输入格式。
3. Adaboost分类器训练:使用提取的特征描述子和ground truth,训练Adaboost分类器。在训练过程中,需要选择合适的弱分类器,并对每个弱分类器进行权重调整,以提高分类器的性能。
首先,我们需要定义一个弱分类器的模板,可以使用简单的if-else语句实现,比如:
```
def weak_classifier(feature_value, threshold, polarity):
if (polarity == 1 and feature_value > threshold) or (polarity == -1 and feature_value <= threshold):
return 1
else:
return -1
```
然后,我们需要定义一个Adaboost分类器类,其中包括弱分类器的训练和分类方法,以及Adaboost分类器的训练和分类方法,可以使用Python实现,比如:
```
class Adaboost:
def __init__(self, T=100):
self.T = T
self.alpha = []
self.classifiers = []
def train_weak_classifier(self, features, labels, weights):
best_error = float('inf')
best_threshold = 0
best_polarity = 1
for feature_idx in range(features.shape[1]):
feature_values = features[:, feature_idx]
thresholds = np.unique(feature_values)
for threshold in thresholds:
for polarity in [-1, 1]:
pred_labels = np.array([weak_classifier(feature_values[i], threshold, polarity) for i in range(features.shape[0])])
error = np.sum(weights[labels != pred_labels])
if error < best_error:
best_error = error
best_threshold = threshold
best_polarity = polarity
return best_error, best_threshold, best_polarity
def train(self, features, labels):
weights = np.ones(features.shape[0]) / features.shape[0]
for t in range(self.T):
error, threshold, polarity = self.train_weak_classifier(features, labels, weights)
alpha = 0.5 * np.log((1 - error) / error)
self.alpha.append(alpha)
self.classifiers.append((threshold, polarity))
pred_labels = np.array([weak_classifier(features[i], threshold, polarity) for i in range(features.shape[0])])
weights *= np.exp(-alpha * labels * pred_labels)
weights /= np.sum(weights)
def classify(self, features):
pred_labels = np.zeros(features.shape[0])
for t in range(self.T):
threshold, polarity = self.classifiers[t]
alpha = self.alpha[t]
pred_labels += alpha * np.array([weak_classifier(features[i], threshold, polarity) for i in range(features.shape[0])])
return np.sign(pred_labels)
```
4. 目标检测:对于新的图像,使用训练好的分类器进行目标检测。首先对图像进行滑动窗口扫描,对每个窗口提取特征描述子,并使用训练好的分类器进行分类。如果分类结果为目标,则将该窗口标记为检测结果。
我们可以使用滑动窗口的方法来遍历整张图像,对每个窗口提取特征描述子,并使用训练好的Adaboost分类器进行分类,判断是否为目标。可以使用以下代码实现:
```
def sliding_window(image, step_size, window_size):
for y in range(0, image.shape[0] - window_size[1], step_size):
for x in range(0, image.shape[1] - window_size[0], step_size):
yield (x, y, image[y:y + window_size[1], x:x + window_size[0]])
def detect_objects(image, classifier, window_size, step_size, threshold):
detections = []
for (x, y, window) in sliding_window(image, step_size, window_size):
feature = extract_feature(window)
score = classifier.classify(feature)
if score > threshold:
detections.append((x, y, x + window_size[0], y + window_size[1]))
return detections
```
其中,`window_size`是窗口的大小,`step_size`是滑动窗口的步长,`threshold`是分类阈值,可以根据实际情况进行调整。
5. 检测结果评估:将检测结果与ground truth进行比对,计算准确率、召回率和F1值等指标,评估算法的性能。可以使用以下代码实现:
```
def evaluate(detections, ground_truth, overlap_threshold):
true_positives = 0
false_positives = 0
false_negatives = 0
for detection in detections:
detected = False
for truth in ground_truth:
overlap = compute_overlap(detection, truth)
if overlap >= overlap_threshold:
detected = True
break
if detected:
true_positives += 1
else:
false_positives += 1
false_negatives = len(ground_truth) - true_positives
precision = true_positives / (true_positives + false_positives)
recall = true_positives / (true_positives + false_negatives)
f1_score = 2 * (precision * recall) / (precision + recall)
return precision, recall, f1_score
def compute_overlap(rect1, rect2):
x1 = max(rect1[0], rect2[0])
y1 = max(rect1[1], rect2[1])
x2 = min(rect1[2], rect2[2])
y2 = min(rect1[3], rect2[3])
if x1 >= x2 or y1 >= y2:
return 0.0
else:
area1 = (rect1[2] - rect1[0]) * (rect1[3] - rect1[1])
area2 = (rect2[2] - rect2[0]) * (rect2[3] - rect2[1])
overlap_area = (x2 - x1) * (y2 - y1)
return overlap_area / (area1 + area2 - overlap_area)
```
其中,`detections`是检测结果,`ground_truth`是ground truth,`overlap_threshold`是重叠阈值,可以根据实际情况进行调整。
希望以上内容对您有所帮助。
相关推荐
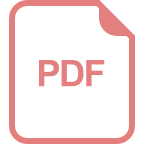
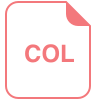








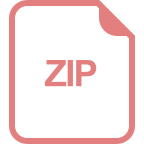
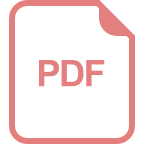
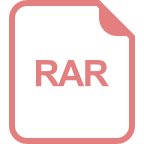