c语言输入从某天的零时整开始计时的总秒数,计算并输出对应的时间
时间: 2024-05-15 20:17:11 浏览: 81
好的,这是一个比较简单的计算题。你需要用输入函数获取从某天的零时整开始计时的总秒数,然后将该秒数转换为对应的时间格式并输出。在C语言中,你可以使用time_t类型来存储从1970年1月1日开始计时的总秒数,然后使用gmtime()函数将该秒数转换为struct tm类型,最后使用strftime()函数将struct tm类型的时间格式化为你需要的字符串格式即可。以下是示例代码:
```c
#include <stdio.h>
#include <time.h>
int main() {
time_t total_seconds;
struct tm *tm_time;
char time_str[100];
printf("请输入从某天的零时整开始计时的总秒数:");
scanf("%ld", &total_seconds);
tm_time = gmtime(&total_seconds);
strftime(time_str, sizeof(time_str), "%Y年%m月%d日 %H时%M分%S秒", tm_time);
printf("对应的时间为:%s\n", time_str);
return 0;
}
```
希望这能够解决你的问题。如果你还有其它问题,请随时问我。
相关问题
c语言实现2 随机抽取一个单词,界面显示该单词的首尾字母以及中间空缺位符,对应的释义,缺失的部分由玩家输入,显示游戏剩余的错误次数,显示出题目的同时开始计时 3 玩家输入完成后,判断其是否正确,当答题剩余3秒时进行提示 4 如果回答正确,则玩家分数加1;如果超时或回答错误,判断为错误,错误剩余次数减1 5 不断重复前面的过程,当剩余错误次数为零时,游戏结束
以下是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define WORD_COUNT 5 // 单词数量
#define MAX_WORD_LEN 20 // 最大单词长度
#define MAX_WRONG_GUESSES 5 // 最大错误次数
#define HIDDEN_CHAR '-' // 隐藏字符
const char *WORDS[WORD_COUNT] = {"apple", "banana", "orange", "grape", "peach"};
const char *DEFINITIONS[WORD_COUNT] = {
"a round fruit with red, green, or yellow skin and a white center",
"a long curved fruit with a yellow skin",
"a round fruit with an orange skin and a sweet juice",
"a small round fruit that is usually purple or green",
"a fruit with a fuzzy yellow or pink skin and a juicy yellow center"
};
int main()
{
srand(time(NULL)); // 初始化随机数生成器
int score = 0; // 玩家得分
int wrong_guesses = 0; // 错误次数
clock_t start_time; // 开始时间
clock_t end_time; // 结束时间
int word_index = rand() % WORD_COUNT; // 随机选择一个单词
const char *word = WORDS[word_index];
const char *definition = DEFINITIONS[word_index];
int len = strlen(word);
char hidden_word[MAX_WORD_LEN + 1];
memset(hidden_word, HIDDEN_CHAR, sizeof(hidden_word));
hidden_word[0] = word[0];
hidden_word[len - 1] = word[len - 1];
printf("Word: %s\n", hidden_word);
printf("Definition: %s\n", definition);
start_time = clock(); // 记录开始时间
while (wrong_guesses < MAX_WRONG_GUESSES)
{
printf("Guess a letter or the whole word: ");
char guess[MAX_WORD_LEN + 1];
scanf("%s", guess);
if (strcmp(guess, word) == 0) // 猜对了整个单词
{
printf("Correct!\n");
score++;
break;
}
else if (strlen(guess) == 1) // 猜对了一个字母
{
int found = 0;
for (int i = 1; i < len - 1; i++)
{
if (word[i] == guess[0])
{
hidden_word[i] = guess[0];
found = 1;
}
}
if (found)
{
printf("Correct!\n");
printf("Word: %s\n", hidden_word);
}
else
{
printf("Wrong!\n");
wrong_guesses++;
printf("You have %d wrong guesses left.\n", MAX_WRONG_GUESSES - wrong_guesses);
}
}
else // 猜错了整个单词
{
printf("Wrong!\n");
wrong_guesses++;
printf("You have %d wrong guesses left.\n", MAX_WRONG_GUESSES - wrong_guesses);
}
if (strcmp(hidden_word, word) == 0) // 猜对了整个单词
{
printf("Correct!\n");
score++;
break;
}
end_time = clock(); // 记录结束时间
double elapsed_time = (double)(end_time - start_time) / CLOCKS_PER_SEC;
if (elapsed_time >= 57) // 剩余3秒
{
printf("Time is running out!\n");
}
}
printf("Game over! Your score is %d.\n", score);
return 0;
}
```
该程序随机选择一个单词,并显示该单词的首尾字母以及中间的空缺字符。玩家需要猜测单词中的字母或整个单词,并在限定的错误次数内猜对。如果猜对了一个字母,程序会显示出现的位置;如果猜对了整个单词,程序会给出正确提示并加分;如果超时或猜错了整个单词,程序会减少错误次数。当错误次数达到最大值时,游戏结束,显示玩家得分。
c语言代码实现 1 使用结构体数据结构 2 随机抽取一个单词,界面显示该单词的首尾字母以及中间空缺位符,对应的释义,缺失的部分由玩家输入,显示游戏剩余的错误次数,显示出题目的同时开始计时 3 玩家输入完成后,判断其是否正确,当答题剩余3秒时进行提示 4 如果回答正确,则玩家分数加1;如果超时或回答错误,判断为错误,错误剩余次数减1 5 不断重复前面的过程,当剩余错误次数为零时,游戏结束
好的,这是一个比较复杂的程序,需要涉及到结构体、字符串、随机数、计时等知识。我会为你梳理一下大致的思路,你可以根据这个思路来编写代码。
1.首先定义一个结构体,包含单词和释义两个成员变量:
```
struct Word {
char word[20];
char meaning[100];
};
```
2.定义一个函数,用于随机抽取一个单词,并显示出题目。在函数内部,先定义一个结构体数组,用于存储所有的单词和释义。然后使用随机数生成一个下标,随机选取一个单词,并将其首尾字母显示出来,中间用下划线代替。同时,记录下正确答案。
```
void showQuestion(struct Word words[], int length, char* answer) {
srand(time(NULL)); // 初始化随机数种子
int index = rand() % length; // 生成一个随机下标
printf("请猜测单词的意思:\n");
printf("%c", words[index].word[0]); // 显示首字母
for (int i = 1; i < strlen(words[index].word) - 1; i++) {
printf("_"); // 显示中间的下划线
}
printf("%c\n", words[index].word[strlen(words[index].word) - 1]); // 显示尾字母
strcpy(answer, words[index].word); // 记录正确答案
}
```
3.定义一个函数,用于判断玩家输入的答案是否正确。在函数内部,比较玩家输入的字符串和正确答案是否相同。如果相同,则返回1,否则返回0。
```
int checkAnswer(char* answer, char* input) {
if (strcmp(answer, input) == 0) {
return 1;
} else {
return 0;
}
}
```
4.定义一个函数,用于显示游戏剩余的错误次数,并开始计时。在函数内部,使用time函数获取当前时间,并记录下来。然后显示游戏剩余的错误次数,并将错误次数减1。最后返回记录的时间。
```
time_t startTimer(int* errorCount) {
time_t start = time(NULL);
printf("剩余错误次数:%d\n", *errorCount);
(*errorCount)--;
return start;
}
```
5.定义一个函数,用于判断是否超时或回答错误。在函数内部,计算当前时间和开始时间的差值,如果差值大于等于3秒,则提示玩家还剩几秒钟。否则,判断玩家的答案是否正确。
```
int checkTimeAndAnswer(char* answer, char* input, time_t start) {
time_t end = time(NULL);
double diff = difftime(end, start);
if (diff >= 3) {
printf("时间快到了,还剩%.0lf秒!\n", 3 - diff);
return 0;
} else {
return checkAnswer(answer, input);
}
}
```
6.最后,定义主函数,不断重复前面的过程,直到错误次数为零。在主函数内部,先定义一个结构体数组,用于存储所有的单词和释义。然后定义一个错误次数,初始化为5。然后进入一个循环,每次循环先调用showQuestion函数随机选取一个单词,并记录正确答案。然后调用startTimer函数开始计时,并显示剩余错误次数,然后等待玩家输入答案。接着调用checkTimeAndAnswer函数判断是否超时或回答错误。如果回答正确,则玩家分数加1。否则,错误次数减1。如果错误次数为零,则游戏结束。
```
int main() {
struct Word words[] = {
{"apple", "苹果"},
{"banana", "香蕉"},
// ...
};
int length = sizeof(words) / sizeof(words[0]);
int errorCount = 5;
int score = 0;
char answer[20];
while (errorCount > 0) {
showQuestion(words, length, answer);
time_t start = startTimer(&errorCount);
char input[20];
scanf("%s", input);
if (checkTimeAndAnswer(answer, input, start)) {
printf("回答正确!\n");
score++;
} else {
printf("回答错误!\n");
}
if (errorCount == 0) {
printf("游戏结束!你的得分是%d\n", score);
}
}
return 0;
}
```
阅读全文
相关推荐
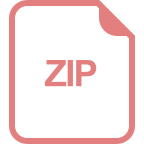
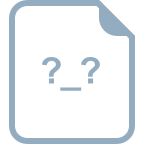
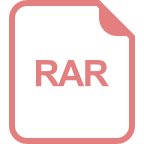
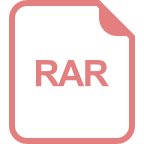
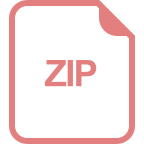
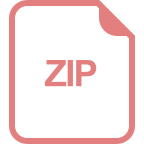
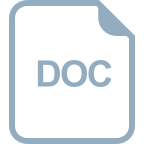


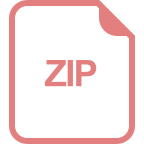
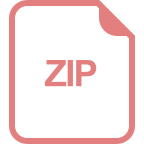
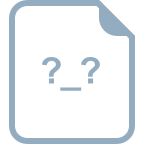
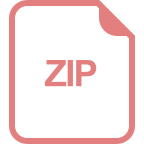