os.system 输出重定向
时间: 2024-06-08 18:12:33 浏览: 16
在Python中,可以使用os.system函数调用系统命令并执行,但是它没有提供直接的输出重定向功能。要实现输出重定向,可以使用sys.stdout重定向标准输出流。具体步骤如下:
1. 首先导入所需的模块:
```
import sys
```
2. 创建一个文件对象,用于保存输出内容:
```
output_file = open("output.txt", "w")
```
3. 将标准输出重定向到文件对象:
```
sys.stdout = output_file
```
4. 调用os.system函数执行系统命令,输出将被重定向到文件中:
```
os.system("command")
```
5. 恢复标准输出:
```
sys.stdout = sys.__stdout__
```
6. 关闭文件对象:
```
output_file.close()
```
通过以上步骤,你可以将os.system函数的输出重定向到指定文件中。请注意,"command"应该被替换为你要执行的系统命令。输出将保存在output.txt文件中,你可以根据需要更改文件名和路径。
相关问题
os.system 如何使用subprocess代替
`os.system` 是一个用于执行系统命令的函数,但它在执行命令时存在一些限制,不够灵活。推荐使用 `subprocess` 模块来替代 `os.system`,因为 `subprocess` 提供了更多的功能和灵活性。
下面是一个示例,展示了如何使用 `subprocess` 模块来代替 `os.system`:
```python
import subprocess
# 使用 subprocess.run 执行命令
result = subprocess.run("ls -l", shell=True, capture_output=True, text=True)
print(result.stdout)
# 使用 subprocess.Popen 执行命令,并获取输出
process = subprocess.Popen("ls -l", shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print(stdout)
```
在上述示例中,我们使用了两种常见的替代方案。
首先,我们使用 `subprocess.run` 函数来执行命令,并捕获其输出。`subprocess.run` 接受多个参数,其中 `shell=True` 表示在 shell 环境中执行命令,`capture_output=True` 表示捕获命令的标准输出和错误输出,`text=True` 表示以文本形式返回输出结果。`result.stdout` 属性保存了命令的标准输出结果。
其次,我们使用 `subprocess.Popen` 类来执行命令,并通过 `communicate` 方法获取命令的输出。`subprocess.Popen` 接受多个参数,其中 `shell=True` 表示在 shell 环境中执行命令,`stdout=subprocess.PIPE` 和 `stderr=subprocess.PIPE` 表示将标准输出和错误输出重定向到管道,`text=True` 表示以文本形式返回输出结果。`process.communicate()` 方法返回一个元组,其中包含了命令的标准输出和错误输出。
使用 `subprocess` 模块可以更好地控制和处理命令的执行,并且提供了更多的功能选项。根据你的需求,可以选择适合的方法来代替 `os.system`。
希望这个示例能帮助你理解如何使用 `subprocess` 来替代 `os.system`!
result = os.system(order)如何输出到指定文件中
`os.system` 函数在执行系统命令时,不会将命令的输出信息保存下来,因此我们需要使用操作系统的重定向功能将命令的输出信息输出到指定文件中。具体方法如下:
```python
import os
# 打开文件,以追加方式写入
with open('output.txt', 'a') as f:
# 执行命令并将输出重定向到文件中
os.system('command > output.txt 2>&1')
```
在上面的代码中,我们使用了操作系统的重定向符号 `>` 将命令的标准输出重定向到文件 `output.txt` 中,`2>&1` 则表示将标准错误输出也重定向到标准输出。因此,所有的输出信息都会被写入到文件中。
注意,这种方法只能将命令的输出信息输出到文件中,无法获取命令的返回值。如果需要获取命令的返回值,可以使用 `subprocess` 模块中的 `check_output` 函数。
相关推荐
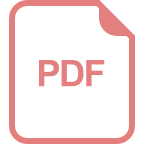
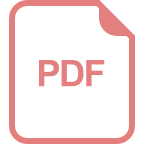
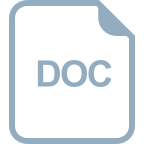












