自己想一个需求不要太复杂-- 让AI实现下, 功能,单元测试,注释,功能说明,解决报错
时间: 2024-10-10 19:04:21 浏览: 15
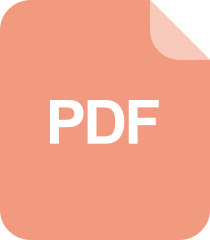
你一定想不到,实现一个AI自动化测试工具就这么简单!
好的,让我们设计一个简单的文本计数器应用。这个应用接收一段字符串作为输入,然后返回其中单词的数量。我们首先会创建一个名为`WordCounter`的简单类,并包含两个方法:一个用于计算单词数,另一个用于处理用户输入并调用这个方法。
```python
# WordCounter.py
class WordCounter:
"""
A class that counts the number of words in a given input string.
Attributes:
None (all functionality is within methods)
"""
def count_words(self, text):
"""
Calculate the number of words in the provided text.
Args:
text (str): The input text to count words from.
Returns:
int: The total word count.
"""
# Remove punctuation and split by whitespace
words = text.translate(str.maketrans('', '', string.punctuation)).split()
# Return the length of the list (number of words)
return len(words)
def process_input(self, user_text):
"""
Takes user input, removes punctuation, counts words, and returns the result.
Args:
user_text (str): The user's input to be counted.
Returns:
int: The word count or an error message if input is empty or not a string.
"""
try:
# Check if input is valid
if isinstance(user_text, str) and user_text.strip():
return self.count_words(user_text)
else:
return "Error: Please enter a non-empty string."
except Exception as e:
return f"Error: {str(e)}"
# Unit test example
import unittest
from WordCounter import WordCounter
class TestWordCounter(unittest.TestCase):
def setUp(self):
self.word_counter = WordCounter()
def test_count_words(self):
self.assertEqual(self.word_counter.count_words("Hello, world!"), 2)
self.assertEqual(self.word_counter.count_words(""), 0)
self.assertEqual(self.word_counter.count_words("This is a test."), 4)
def test_process_input(self):
self.assertEqual(self.word_counter.process_input("Hello, world!"), 2)
self.assertEqual(self.word_counter.process_input(""), "Error: Please enter a non-empty string.")
self.assertIsInstance(self.word_counter.process_input(123), str)
if __name__ == "__main__":
unittest.main()
```
阅读全文
相关推荐
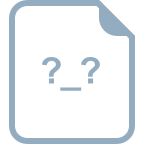
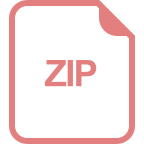
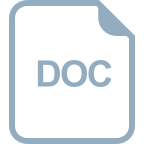
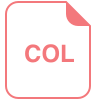
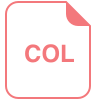
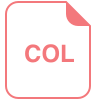
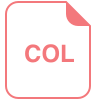
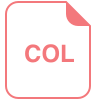
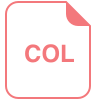
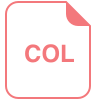
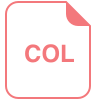
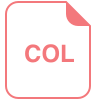
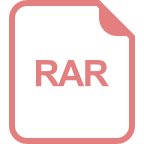
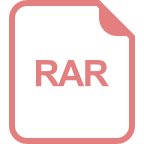
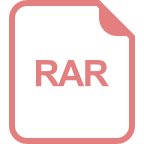
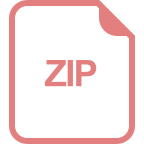