python 搭建的xgboost模型使用sequential进行调参
时间: 2024-10-13 20:06:12 浏览: 29
在Python中,XGBoost是一个强大的梯度提升库,用于训练梯度增强决策树。如果你想通过Sequential(一种常用的网格搜索和随机搜索工具,通常在Scikit-Learn库中的`sklearn.model_selection.SequentialSearchCV`)来调优XGBoost模型,你需要遵循以下步骤:
1. **导入所需库**:
```python
from sklearn.model_selection import SequentialSearchCV
from xgboost import XGBClassifier
from sklearn.datasets import load_iris
```
2. **加载数据集**:
```python
iris = load_iris()
X = iris.data
y = iris.target
```
3. **准备参数空间**:
创建一个包含可能参数值的字典,例如学习率、最大深度等。你可以尝试不同的范围:
```python
param_grid = {
'learning_rate': [0.05, 0.1, 0.2],
'max_depth': [3, 4, 5],
'n_estimators': [100, 200, 300],
'colsample_bytree': [0.6, 0.8, 1.0]
# ...其他可能的参数
}
```
4. **创建XGBoost分类器**:
```python
model = XGBClassifier(objective='multi:softmax', num_class=3) # 假设是多类别问题,num_class为类别数
```
5. **使用SequentialSearchCV进行调优**:
```python
search = SequentialSearchCV(
estimator=model,
param_distributions=param_grid,
n_iter=10, # 进行多少次迭代,默认为30
scoring='accuracy',
cv=5, # 使用交叉验证次数
)
```
6. **开始搜索并获取最佳参数组合**:
```python
search.fit(X, y)
best_params = search.best_params_
```
7. **评估最佳模型**:
```python
best_model = search.best_estimator_
best_score = search.best_score_
```
阅读全文
相关推荐
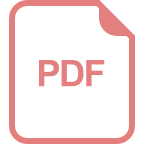
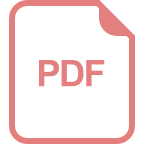
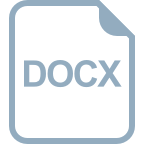
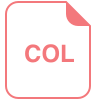
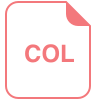
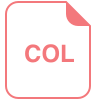
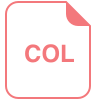
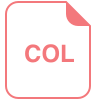
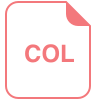
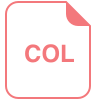
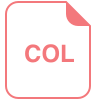
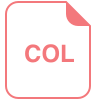
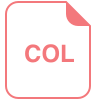
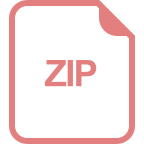