LinkedBlockingQueue poll
时间: 2023-09-04 22:14:55 浏览: 146
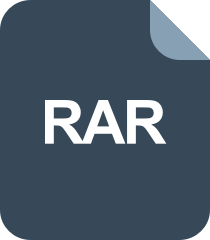
linkedblockingqueue
LinkedBlockingQueue的`poll()`方法用于获取并移除队首的元素,如果队列为空则返回null。
该方法有两个重载形式:
1. `poll()`: 无参数的`poll()`方法会立即返回队首的元素,如果队列为空则返回null。
2. `poll(long timeout, TimeUnit unit)`: 带有超时参数的`poll()`方法会在指定的时间内等待获取队首的元素。如果在超时时间内队列为空,则返回null。
下面是一个简单的示例代码,演示了如何使用`poll()`方法获取并移除队首的元素:
```java
import java.util.concurrent.LinkedBlockingQueue;
public class LinkedBlockingQueueExample {
public static void main(String[] args) {
LinkedBlockingQueue<Integer> queue = new LinkedBlockingQueue<>();
// 添加元素到队列
queue.add(1);
queue.add(2);
queue.add(3);
// 获取并移除队首的元素
Integer firstElement = queue.poll();
System.out.println("First Element: " + firstElement);
// 获取并移除队首的元素(带有超时参数)
try {
Integer elementWithTimeout = queue.poll(1, TimeUnit.SECONDS);
System.out.println("Element with Timeout: " + elementWithTimeout);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
输出结果:
```
First Element: 1
Element with Timeout: 2
```
在示例中,首先我们向队列中添加了三个元素。然后使用`poll()`方法获取并移除队首的元素,第一个元素为1。接着使用带有超时参数的`poll()`方法,等待1秒钟获取并移除队首的元素,此时队列中还剩下一个元素2。
需要注意的是,如果队列为空而调用`poll()`方法,则会立即返回null,或者在指定的超时时间内等待元素并返回null。
阅读全文
相关推荐
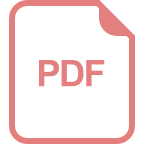
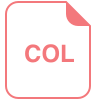












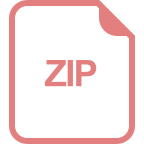