java线程安全的单例模式代码
时间: 2023-11-28 21:42:46 浏览: 93
以下是Java线程安全的单例模式代码:
```
public class Singleton {
private static volatile Singleton instance = null;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
```
这个单例模式代码使用了双重检查锁定(double-checked locking)来确保线程安全。在第一次检查中,如果实例已经存在,那么就直接返回实例。如果实例不存在,那么就进入同步块,在同步块中再次检查实例是否存在,如果不存在,那么就创建实例。使用volatile关键字可以确保多线程环境下的可见性和有序性。
相关问题
java枚举单例模式代码
下面是Java枚举单例模式的代码:
```java
public enum Singleton {
INSTANCE;
public void testMethod() {
System.out.println("执行了单例类的方法");
}
}
```
使用枚举实现单例模式的好处是,枚举类只会被加载一次,保证了线程安全和单例的唯一性。同时,枚举类也可以防止反序列化和反射破坏单例。
要使用这个单例类,只需要调用`Singleton.INSTANCE`即可,这个实例是全局唯一的。例如:
```java
Singleton.INSTANCE.testMethod();
System.out.println(Singleton.INSTANCE);
```
这段代码会输出“执行了单例类的方法”和“INSTANCE”。
懒汉单例模式代码Java实现
以下是懒汉单例模式的 Java 代码实现:
```
public class LazySingleton {
private static LazySingleton instance;
private LazySingleton() {}
public static synchronized LazySingleton getInstance() {
if (instance == null) {
instance = new LazySingleton();
}
return instance;
}
}
```
这是一个线程安全的懒汉单例模式,通过 getInstance() 方法获取实例。
阅读全文
相关推荐
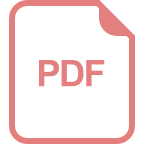
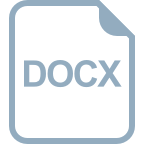
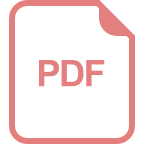
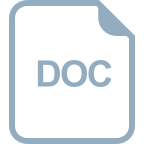
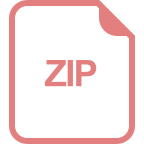
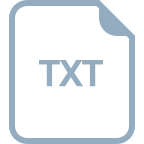
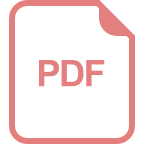
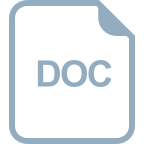
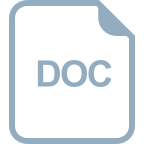
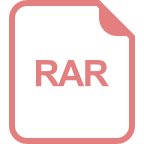
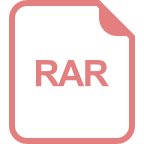
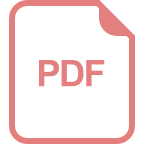
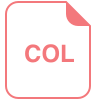
