python 创建多层文件夹和文件
时间: 2023-09-04 11:01:53 浏览: 235
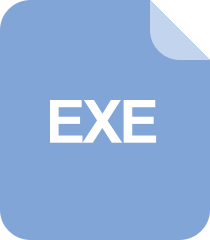
快速建立多层文件夹
Python可以用os模块来创建多层文件夹和文件。利用os模块中的函数,我们可以很方便地实现这个功能。
首先,我们需要导入os模块。
```
import os
```
要创建文件夹,可以使用os.makedirs()函数,该函数支持创建多层文件夹。我们可以指定一个路径,如果在路径中的文件夹不存在,就会被自动创建。
```
os.makedirs("path/to/folder")
```
上述代码中的"path/to/folder"是要创建的文件夹路径,可以根据实际情况进行修改。
要创建文件,我们可以使用open()函数来创建文件,并指定文件路径和文件名。
```
file_path = "path/to/file.txt"
file = open(file_path, "w")
```
上述代码中的"path/to/file.txt"是要创建的文件路径和文件名,可以根据实际情况进行修改。另外,"w"表示以写入模式打开文件。
最后,不要忘记在完成文件操作后关闭文件,可以使用file.close()来关闭文件。
上述内容是使用Python创建多层文件夹和文件的简单示例。在实际应用中,我们可以根据需要进行调整和扩展。
阅读全文
相关推荐
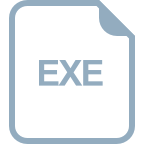
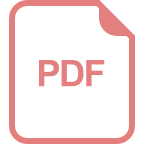
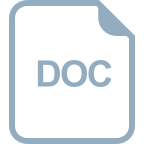
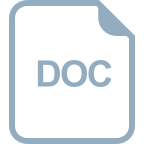

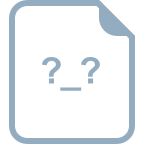
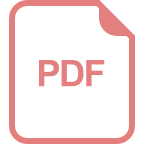
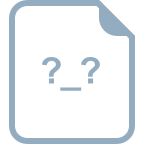
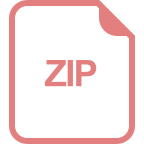
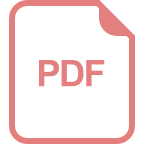
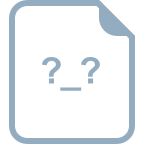
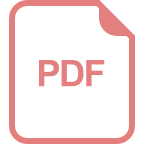
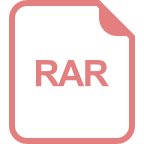
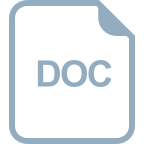
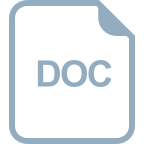
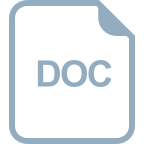
