springboot 复杂邮件发送
时间: 2023-12-13 22:33:42 浏览: 118
以下是使用Spring Boot发送复杂邮件的步骤:
1.在pom.xml文件中添加spring-boot-starter-mail依赖项。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
<version>2.2.6.RELEASE</version>
</dependency>
```
2.在application.properties文件中配置邮件服务器的相关信息。
```properties
spring.mail.host=smtp.example.com
spring.mail.port=587
spring.mail.username=yourusername
spring.mail.password=yourpassword
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.starttls.enable=true
```
3.创建一个邮件服务类,该类将使用JavaMailSender发送邮件。
```java
@Service
public class EmailService {
@Autowired
private JavaMailSender mailSender;
public void sendEmailWithAttachment(String to, String subject, String text, String pathToAttachment) throws MessagingException {
MimeMessage message = mailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setTo(to);
helper.setSubject(subject);
helper.setText(text);
FileSystemResource file = new FileSystemResource(new File(pathToAttachment));
helper.addAttachment("Attachment", file);
mailSender.send(message);
}
}
```
4.在控制器中使用邮件服务类发送邮件。
```java
@RestController
public class EmailController {
@Autowired
private EmailService emailService;
@PostMapping("/sendEmail")
public ResponseEntity<String> sendEmail(@RequestParam String to, @RequestParam String subject, @RequestParam String text, @RequestParam String pathToAttachment) {
try {
emailService.sendEmailWithAttachment(to, subject, text, pathToAttachment);
return ResponseEntity.ok("Email sent successfully.");
} catch (MessagingException e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Error sending email: " + e.getMessage());
}
}
}
```
阅读全文
相关推荐
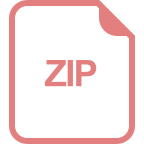
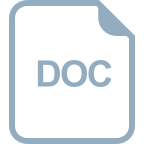
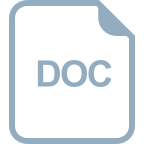
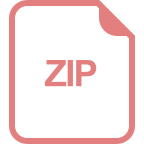
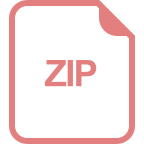
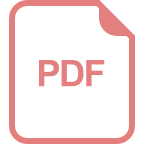
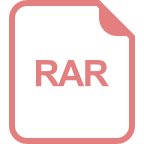
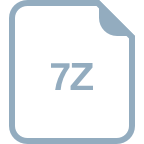
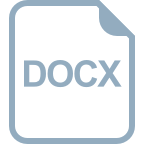
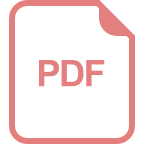
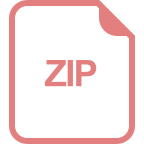
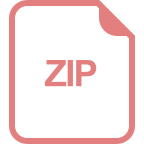
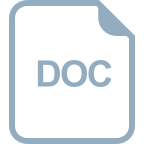
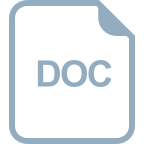
