制作一个通讯录,利用顺序存储结构(或者链式存储结构)实现电话本的基本操作,比如通讯录的建立、插入记录、删除记录、修改记录、查找记录和输出通讯录等操作。(功能不限于所述这些)使用C++实现;
时间: 2024-10-21 21:08:19 浏览: 38
在C++中,我们可以使用两种数据结构来制作一个简单的通讯录:顺序存储(数组或动态数组)和链式存储(链表)。这里我们分别讲解这两种情况。
**1. 使用顺序存储(数组)**
```cpp
#include <iostream>
using namespace std;
// 定义一个联系人结构体
struct Contact {
string name;
string phone_number;
};
// 序列化通讯录
class AddressBook {
private:
int size;
Contact contacts[100]; // 这里假设最大容量为100,可根据实际需要调整
public:
AddressBook() : size(0) {}
// 插入记录
void insert(const Contact& contact) {
if (size == 100) {
cout << "通讯录已满,无法添加新的联系人。\n";
return;
}
contacts[size++] = contact;
}
// 删除记录
bool deleteContact(int index) {
if (index < 0 || index >= size) {
cout << "无效的索引。\n";
return false;
}
for (int i = index; i < size - 1; ++i) {
contacts[i] = contacts[i + 1];
}
--size;
return true;
}
// 修改记录
bool modifyContact(int index, const Contact& newContact) {
if (index < 0 || index >= size) {
cout << "无效的索引。\n";
return false;
}
contacts[index] = newContact;
return true;
}
// 查找记录
bool findContact(string name, Contact& foundContact) {
for (int i = 0; i < size; ++i) {
if (contacts[i].name == name) {
foundContact = contacts[i];
return true;
}
}
return false;
}
// 输出通讯录
void print() {
for (int i = 0; i < size; ++i) {
cout << "姓名: " << contacts[i].name << ", 电话号码: " << contacts[i].phone_number << endl;
}
}
};
int main() {
AddressBook book;
// 实现其他基本操作...
return 0;
}
```
**2. 使用链式存储(链表)**
```cpp
#include <iostream>
using namespace std;
// 链表节点
struct ContactNode {
string name;
string phone_number;
ContactNode* next;
};
class AddressBookLinkedList {
private:
ContactNode* head;
public:
AddressBookLinkedList() : head(nullptr) {}
// ... 同上,实现相似的操作,但是插入、删除、查找和输出都需要遍历链表,效率较低...
// 插入节点
void insert(const Contact& contact) {
// 创建新节点并链接到列表
auto newNode = new ContactNode{contact.name, contact.phone_number, nullptr};
if (!head) {
head = newNode;
} else {
ContactNode* current = head;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
}
// ... 省略其他操作的实现...
};
int main() {
AddressBookLinkedList bookList;
// 实现其他基本操作...
return 0;
}
```
注意:上述代码只提供了一个基础框架,具体的实现细节可能会有所不同。你可以根据需要选择适合的数据结构,并确保处理好内存管理(如在链表版本中避免内存泄漏)。在实际应用中,还要考虑错误处理和用户交互等问题。如果你有更多关于链表和顺序存储的具体操作疑问,请提问:
阅读全文
相关推荐
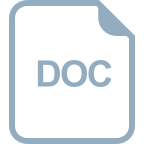
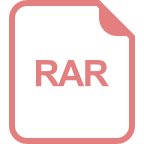
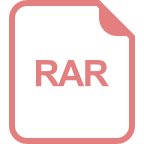
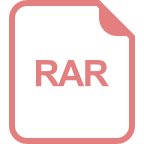
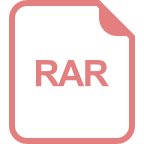
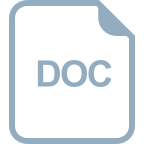
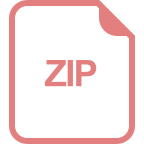
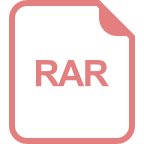
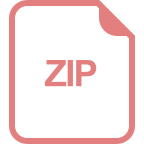
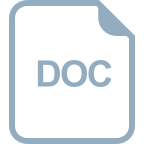
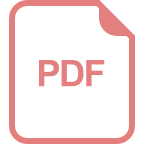
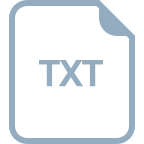
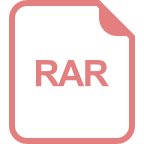
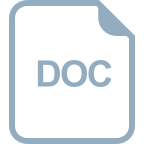
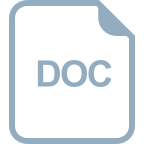
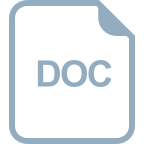
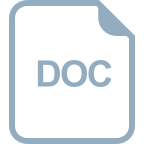
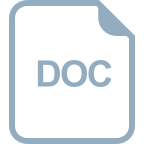

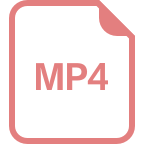