C语言顺序查找返回x多次出现的下标值
时间: 2024-12-19 11:15:33 浏览: 4
在C语言中,如果你想要顺序查找数组中某个元素`x`可能出现的所有下标值,并非内置功能,你需要手动遍历数组。下面是一个简单的示例,这个函数会在数组中查找并返回所有等于`x`的位置:
```c
#include <stdio.h>
void find_all_indices(int arr[], int n, int x, int indices[]) {
int i = 0;
for (i = 0; i < n; i++) {
if (arr[i] == x) {
indices[i] = i; // 当找到匹配项时,将当前索引添加到结果数组
} else if (i > 0 && arr[i] == arr[i - 1]) { // 如果发现连续的x,记录它们的起始位置
if (indices[i - 1] != -1) {
indices[indices[i - 1]]++; // 更新上一次找到的x之后的第一个x的索引
} else {
indices[i] = i - 1; // 初始化新连续段的起始位置
}
}
}
}
int main() {
int arr[] = {1, 2, 3, 4, 2, 5, 2};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 2;
int indices[n]; // 存储结果的数组,默认初始化为-1表示未找到
find_all_indices(arr, n, x, indices);
printf("Element %d appears at the following indices: ", x);
for (int i = 0; i < n; i++) {
if (indices[i] != -1) {
printf("%d, ", indices[i]);
}
}
return 0;
}
```
注意:此代码仅适用于已排序的数组,因为它是依赖于前一个元素是否相等来判断是否连续出现的。如果输入数组不是有序的,结果可能会不准确。
阅读全文
相关推荐




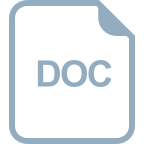












