java 实现以下方法:public void readExcel(String scanPath, String date, String tableName, String insertType), 先判断如果date不为"",则在scanPath路径后拼接一个date,然后开始扫描路径下所有excel文件,读取excel的数据,将数据插入tableName表中,若insertType为"add",则直接插入,若insertType为"all",则清除表数据后再插入
时间: 2023-12-03 17:46:33 浏览: 91
以下是 Java 实现 readExcel 方法的代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Iterator;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFDateUtil;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelReader {
public void readExcel(String scanPath, String date, String tableName, String insertType) throws Exception {
// If date is not empty, append it to the scan path
if (!date.equals("")) {
scanPath += File.separator + date;
}
// Create a connection to the database
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "root", "password");
// If insertType is "all", clear the table before inserting data
if (insertType.equalsIgnoreCase("all")) {
PreparedStatement deleteStmt = conn.prepareStatement("DELETE FROM " + tableName);
deleteStmt.executeUpdate();
deleteStmt.close();
}
// Iterate through all Excel files in the scan path
File folder = new File(scanPath);
File[] files = folder.listFiles();
for (File file : files) {
if (file.isFile() && (file.getName().endsWith(".xls") || file.getName().endsWith(".xlsx"))) {
FileInputStream inputStream = new FileInputStream(file);
Sheet sheet = null;
if (file.getName().endsWith(".xls")) {
HSSFWorkbook workbook = new HSSFWorkbook(inputStream);
sheet = workbook.getSheetAt(0);
} else if (file.getName().endsWith(".xlsx")) {
XSSFWorkbook workbook = new XSSFWorkbook(inputStream);
sheet = workbook.getSheetAt(0);
}
Iterator<Row> rowIterator = sheet.iterator();
while (rowIterator.hasNext()) {
Row row = rowIterator.next();
if (row.getRowNum() == 0) {
continue; // Skip the header row
}
Iterator<Cell> cellIterator = row.cellIterator();
StringBuilder sqlBuilder = new StringBuilder("INSERT INTO " + tableName + " VALUES (");
while (cellIterator.hasNext()) {
Cell cell = cellIterator.next();
int cellType = cell.getCellType();
if (cellType == Cell.CELL_TYPE_NUMERIC && HSSFDateUtil.isCellDateFormatted(cell)) {
sqlBuilder.append("'" + new java.sql.Date(cell.getDateCellValue().getTime()) + "'");
} else {
sqlBuilder.append("'" + cell.toString() + "'");
}
if (cellIterator.hasNext()) {
sqlBuilder.append(", ");
}
}
sqlBuilder.append(")");
PreparedStatement insertStmt = conn.prepareStatement(sqlBuilder.toString());
insertStmt.executeUpdate();
insertStmt.close();
}
inputStream.close();
}
}
// Close the database connection
conn.close();
}
}
```
在这个实现中,我们使用 Apache POI 库来读取 Excel 文件中的数据。首先,我们判断 date 参数是否为空,如果不为空,则将其附加到 scanPath 参数的末尾。然后,我们创建一个连接到数据库的对象 conn,并检查 insertType 参数的值。如果 insertType 为 "all",则使用 PreparedStatement 对象执行 DELETE 语句来清空表中的数据。接下来,我们遍历 scanPath 目录下的所有 Excel 文件,并使用 FileInputStream 对象读取每个文件的内容。根据文件扩展名的不同,我们使用 HSSFWorkbook 或 XSSFWorkbook 对象来解析文件。然后,我们遍历每个文件中的所有行和单元格,并使用 StringBuilder 对象构造插入语句。最后,我们使用 PreparedStatement 对象执行插入语句。读取完成后,我们关闭连接对象 conn。
阅读全文
相关推荐
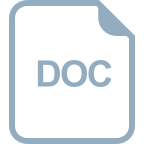
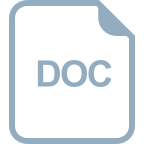
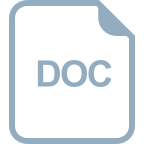
















