如何让C++项目和数据库建立连接
时间: 2024-10-14 12:08:31 浏览: 31
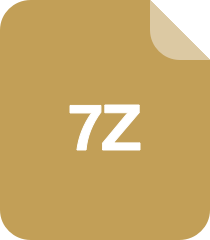
基于C++的MySQL数据库连接池源代码
在C++项目中与数据库建立连接通常需要借助数据库驱动程序或ORM(Object-Relational Mapping)库。以下是基本步骤:
1. **选择数据库驱动**:
- 对于SQL数据库,可以选择MySQL Connector/C++、PostgreSQL libpq、SQLite 3.x等官方提供的C++接口,或者像Qt SQL、ODBC等跨平台库。
2. **安装库和头文件**:
- 根据所选驱动,确保已正确安装库文件,并将头文件添加到项目的包含路径中。
3. **连接设置**:
- 编程时,创建一个数据库连接对象并初始化它,提供必要的参数如主机名、端口、用户名、密码以及数据库名称。
```cpp
#include <sql.h>
#include <mysql_driver.h>
#include <cppconn/driver.h>
#include <cppconn/statement.h>
sql::Driver *driver;
sql::Connection *con;
// 初始化连接
try {
driver = sql::get_mysql_driver_instance();
con = driver->connect("tcp://localhost:3306", "username", "password");
} catch (sql::SQLException &e) {
std::cerr << "# ERR: SQLException caught during connect\n";
std::cerr << "# ERR: " << e.what() << endl;
}
```
4. **执行查询**:
使用`Statement`对象执行SQL命令,获取数据,或者更新数据库。
5. **处理结果**:
执行完毕后,关闭连接和 statement,释放资源。
```cpp
sql::Statement *stmt;
try {
stmt = con->createStatement();
// 执行查询
stmt->execute("SELECT * FROM table_name");
// 读取结果
while (stmt->next()) {
// 处理每一行数据
}
} catch (sql::SQLException &e) {
std::cerr << "# ERR: SQLException caught during query\n";
std::cerr << "# ERR: " << e.what() << "\n";
}
// 关闭资源
delete stmt;
delete con;
```
阅读全文
相关推荐
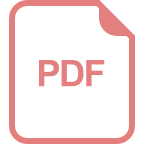
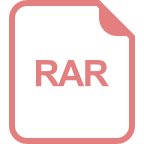
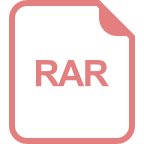
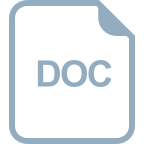
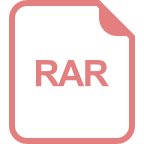
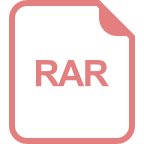
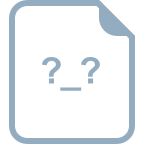
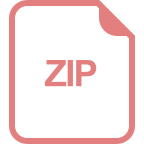
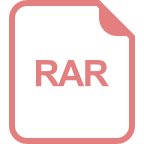
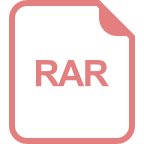
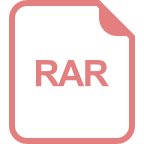
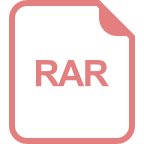
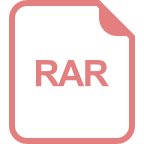
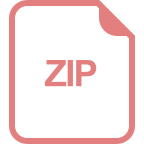
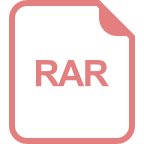
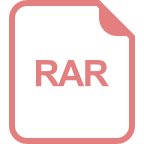
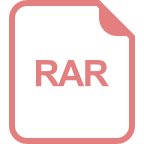
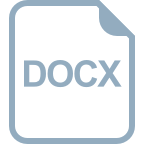