基于springcloud的登录注册功能
时间: 2024-01-10 21:43:43 浏览: 132
基于Spring Cloud的登录注册功能可以使用Spring Security和OAuth2进行实现。
首先,您需要添加以下依赖项到您的pom.xml文件中:
```
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-oauth2</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-security</artifactId>
</dependency>
```
然后,您需要配置Spring Security和OAuth2。以下是一个简单的配置示例:
```
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Autowired
private PasswordEncoder passwordEncoder;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder);
}
@Bean
public JwtAccessTokenConverter jwtAccessTokenConverter() {
JwtAccessTokenConverter converter = new JwtAccessTokenConverter();
converter.setSigningKey("secret");
return converter;
}
@Bean
public TokenStore tokenStore() {
return new JwtTokenStore(jwtAccessTokenConverter());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable().authorizeRequests()
.antMatchers("/oauth/**", "/login/**", "/logout/**").permitAll()
.anyRequest().authenticated()
.and().formLogin().permitAll()
.and().logout().permitAll();
}
@Bean
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
}
```
上述代码将配置Spring Security和OAuth2,其中:
- `@EnableWebSecurity`注释启用Web安全性。
- `@EnableGlobalMethodSecurity(prePostEnabled = true)`允许使用@PreAuthorize和@PostAuthorize注释来实现方法级别的安全性。
- `configure(AuthenticationManagerBuilder auth)`方法配置使用的用户详细信息服务和密码编码器。
- `JwtAccessTokenConverter`和`TokenStore` bean将用于生成和验证JWT令牌。
- `configure(HttpSecurity http)`方法配置允许所有人访问OAuth2和登录/注销端点,并要求所有其他请求都需要进行身份验证。
- `authenticationManagerBean()`方法将创建一个AuthenticationManager bean,该bean将在OAuth2授权服务器配置中使用。
接下来,您需要创建一个OAuth2授权服务器。以下是一个简单的示例:
```
@Configuration
@EnableAuthorizationServer
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
@Autowired
private AuthenticationManager authenticationManager;
@Autowired
private UserDetailsService userDetailsService;
@Autowired
private JwtAccessTokenConverter jwtAccessTokenConverter;
@Autowired
private TokenStore tokenStore;
@Value("${security.oauth2.client.client-id}")
private String clientId;
@Value("${security.oauth2.client.client-secret}")
private String clientSecret;
@Value("${security.oauth2.client.access-token-validity-seconds}")
private int accessTokenValiditySeconds;
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory().withClient(clientId)
.secret(passwordEncoder().encode(clientSecret))
.authorizedGrantTypes("password", "refresh_token")
.accessTokenValiditySeconds(accessTokenValiditySeconds)
.scopes("read", "write");
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.authenticationManager(authenticationManager)
.accessTokenConverter(jwtAccessTokenConverter)
.userDetailsService(userDetailsService)
.tokenStore(tokenStore);
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
上述代码将配置OAuth2授权服务器,其中:
- `@EnableAuthorizationServer`注释启用授权服务器。
- `configure(ClientDetailsServiceConfigurer clients)`方法配置客户端详细信息,包括客户端ID和密码。
- `configure(AuthorizationServerEndpointsConfigurer endpoints)`方法配置授权服务器端点,包括身份验证管理器,用户详细信息服务,JWT令牌转换器和令牌存储器。
- `passwordEncoder()` bean将用于加密客户端密码。
最后,您需要创建一个用户详细信息服务来返回用户信息。以下是一个示例:
```
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
// 根据用户名查询用户信息,例如从数据库中查询
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found with username: " + username);
}
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(),
Collections.singleton(new SimpleGrantedAuthority("ROLE_USER")));
}
}
```
上述代码将查询用户信息并返回Spring Security的UserDetails对象。
现在,您可以使用Spring Security和OAuth2创建基于Spring Cloud的登录注册功能。客户端可以通过OAuth2授权服务器获取访问令牌,并使用该令牌访问受保护的资源。
阅读全文
相关推荐
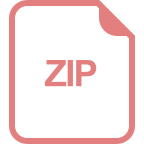
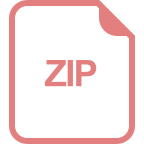
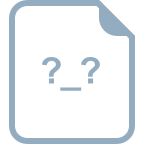
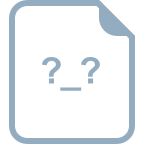
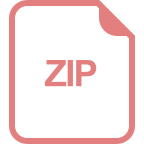
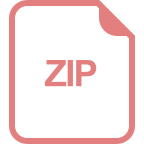
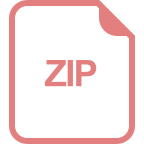
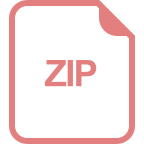
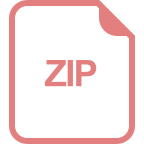
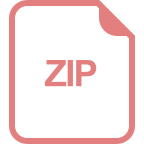
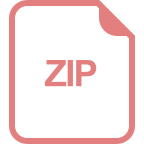
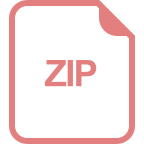
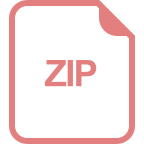
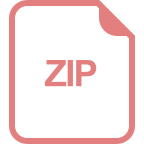
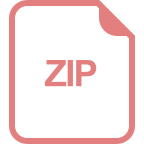
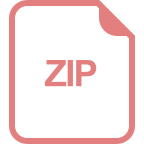