More cats! Fill in this implemention of a class called NoisyCat, which is just like a normal Cat. However, NoisyCat talks a lot -- twice as much as a regular Cat! We may change the implementation of Pet and Cat while testing your code, so make sure you use inheritance correctly.其中class Pet: def init(self, name, owner): self.is_alive = True self.name = name self.owner = owner def eat(self, thing): print(self.name + " ate a " + str(thing) + "!") def talk(self): print(self.name) class Cat(Pet): def init(self, name, owner, lives=9): super().init(name, owner) self.lives = lives def talk(self): print(self.name + " says meow!") def eat(self, thing): super().eat(thing) def lose_life(self): if self.lives > 0: self.lives -= 1 if self.lives == 0: self.is_alive = False else: print(self.name + " has no more lives to lose.")
时间: 2024-03-03 18:51:14 浏览: 114
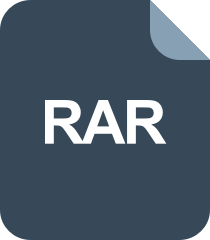
Dogs-vs-Cats.rar_dog vs. cats. ppt_dog_vs_cat_python_深度学习 图片_猫狗

下面是实现 NoisyCat 类的代码:
```
class NoisyCat(Cat):
def talk(self):
print((self.name + " says meow! ") * 2)
```
NoisyCat 类继承自 Cat 类,并重写了 talk() 方法。在 NoisyCat 中,talk() 方法会调用 Cat 中的 talk() 方法,并将其输出内容重复一遍。这样,NoisyCat 对象的 talk() 方法输出的内容就是 Cat 对象的两倍。
阅读全文
相关推荐
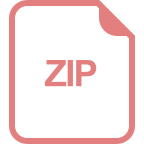
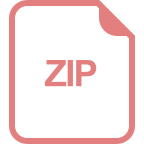

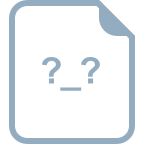
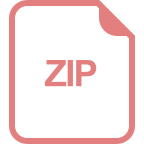
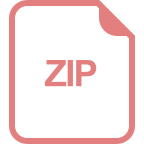
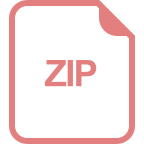
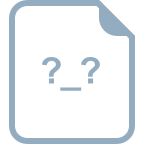
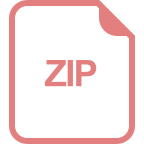
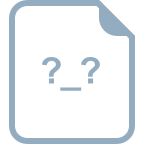
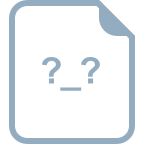
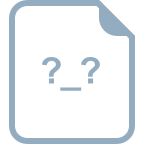
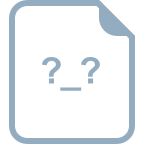



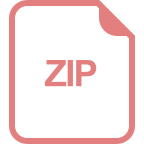
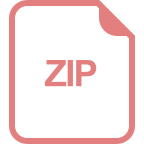
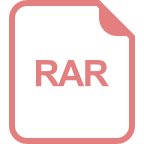