paramiko 实时打印
时间: 2023-08-14 07:07:19 浏览: 50
使用 paramiko 远程执行命令时,需要实时打印远程命令的输出,可以使用 `invoke_shell` 方法来实现。示例代码如下:
```python
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect('hostname', username='root', password='password')
# 打开一个交互式的 shell
channel = ssh.invoke_shell()
# 发送命令
channel.send('ls\n')
# 读取输出
while not channel.recv_ready():
pass
output = channel.recv(1024).decode('utf-8')
print(output)
# 关闭连接
ssh.close()
```
这里的关键是使用 `invoke_shell` 方法打开一个交互式的 shell,然后使用 `send` 方法发送命令,使用 `recv` 方法接收输出。需要注意的是,`recv` 方法是阻塞的,需要等待远程服务器返回数据才能继续执行后面的代码。因此,我们使用一个循环来等待数据的到来。
相关问题
paramiko tail实时打印日志
Paramiko是Python中的SSH客户端,可以使用它来连接到远程服务器并执行命令。如果要实时打印日志,可以使用Paramiko的`invoke_shell`方法来打开一个交互式shell,然后使用`recv`方法来读取实时输出。以下是一个示例代码:
```python
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect('remote_host', username='username', password='password')
stdin, stdout, stderr = ssh.exec_command('tail -f /path/to/logfile')
for line in iter(lambda: stdout.readline(2048), ""):
print(line, end="")
```
以上代码连接到远程主机并打开一个交互式shell,然后执行`tail -f`命令来实时打印日志。`iter(lambda: stdout.readline(2048), "")`是一个无限循环,每次从`stdout`中读取一行输出并打印出来。
需要注意的是,由于`tail -f`命令是一个长时间运行的命令,所以在读取输出时需要设置一个适当的缓冲区大小。在上面的示例中,我们设置缓冲区大小为2048字节。
pycharm 安装paramiko
PyCharm是一款非常强大且开发者喜欢的Python集成开发环境,它将Python开发变得更加轻松。Paramiko是一个Python库,可以用于SSH客户端和服务器端的操作。在PyCharm中安装paramiko非常简单,但有几个步骤需要跟随。
首先,打开PyCharm并在菜单栏中选择“File”,然后选择“Settings”选项。在“Settings”窗口中,选择“Project Interpreter”并单击右上方的加号按钮。在弹出的对话框中选择“Search”,并搜索“paramiko”。在搜索结果中找到“paramiko”并单击“Install Package”按钮。这将开始安装Paramiko。
一旦安装完成,PyCharm会在项目解释器中添加Paramiko软件包。您可以从您的代码中导入它并开始使用它提供的函数和方法。确定安装已完成,请在您的代码中导入Paramiko并开始使用它,例如:
```
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect('hostname', username='username', password='password')
stdin, stdout, stderr = ssh.exec_command('ls -l')
print(stdout.readlines())
ssh.close()
```
这是一个简单的Paramiko示例,将连接到远程服务器并执行Linux命令“ls -l”,然后将其输出打印到控制台。
总而言之,PyCharm在安装Paramiko方面提供了便利,并且只需要几个简单的步骤。安装完成后,您将拥有SSH客户端和服务器端的强大功能,可用于您的Python项目。
相关推荐
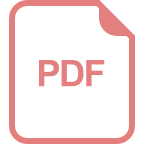
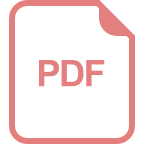
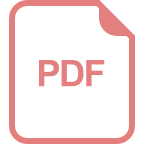












