vue 如何截图iframe
时间: 2023-10-13 10:06:07 浏览: 42
在 Vue 中,可以使用 html2canvas 库来截取 iframe 的内容。具体步骤如下:
1. 安装 html2canvas 库
```
npm install html2canvas --save
```
2. 在需要截取 iframe 内容的组件中引入 html2canvas :
```js
import html2canvas from 'html2canvas';
```
3. 在组件中添加一个用于截取的方法,例如:
```js
methods: {
captureScreenshot() {
const iframe = document.querySelector('iframe'); // 获取需要截取的 iframe 元素
html2canvas(iframe.contentWindow.document.body).then(canvas => { // 使用 html2canvas 进行截取
const imgData = canvas.toDataURL();
// 在这里可以将 imgData 保存或者显示在页面中
});
}
}
```
在这个方法中,我们首先获取需要截取的 iframe 元素,然后通过 html2canvas 将其内容截取为 canvas 对象。最后,我们可以将 canvas 对象转换为图片数据,并进行保存或者显示在页面中。
需要注意的是,由于同源策略的限制,如果 iframe 的内容来自不同的域名,则可能无法截取。
相关问题
vue 截屏 iframe
以下是使用Vue实现截屏iframe的方法:
1.首先需要安装html2canvas库,可以使用npm进行安装:
```shell
npm install html2canvas --save
```
2.在需要截屏的组件中引入html2canvas库:
```javascript
import html2canvas from 'html2canvas'
```
3.在组件中定义一个方法,用于截屏iframe:
```javascript
methods: {
captureScreen() {
// 获取iframe的dom结构
const iframeHtml = this.$refs.iframe.contentWindow
// 获取iframe内容
const iframeBody = iframeHtml.document.getElementsByTagName('body')[0]
// 获取iframe滚动的距离
const iframeScrollY = iframeHtml.document.documentElement.scrollTop
const iframeScrollX = iframeHtml.document.documentElement.scrollLeft
// 将滚动的距离和要截取的宽高传给html2canvas
html2canvas(iframeBody, {
allowTaint: true,
useCORS: true,
width: 812, // TODO 截屏按照1920*1080分辨率下的预览窗口宽高
height: 661,
x: iframeScrollX,
y: iframeScrollY
}).then(canvas => {
// 转成图片,生成图片地址
const imgBase64 = canvas.toDataURL('image/png')
if (imgBase64) {
// 将图片地址赋值给data中的变量
this.imgSrc = imgBase64
} else {
console.error('图片转换出错')
}
})
}
}
```
4.在模板中使用iframe,并在需要截屏的元素上添加ref属性:
```html
<template>
<div>
<iframe ref="iframe" src="http://www.example.com"></iframe>
<button @click="captureScreen">截屏</button>
<img :src="imgSrc" alt="">
</div>
</template>
```
vue-iframe
vue-iframe 是一个Vue的第三方组件,用于在Vue应用中方便地嵌入和操作iframe。它提供了一些功能来处理iframe的加载、跨域通信等问题。
你可以通过以下步骤来使用 vue-iframe:
1. 首先,安装 vue-iframe:
```bash
npm install vue-iframe
```
2. 在需要使用iframe的Vue组件中,引入 vue-iframe 组件,并在组件的 `components` 属性中注册 `Iframe` 组件:
```javascript
import Iframe from 'vue-iframe';
// ...
export default {
// ...
components: {
Iframe
},
// ...
}
```
3. 在模板中使用 vue-iframe 组件来嵌入 iframe:
```html
<template>
<div>
<Iframe :src="iframeSrc" :height="iframeHeight" :width="iframeWidth" @load="handleIframeLoad"></Iframe>
</div>
</template>
```
其中,`:src` 属性是一个动态绑定的属性,可以根据需要设置 iframe 的 src;`:height` 和 `:width` 属性用于设置 iframe 的高度和宽度;`@load` 是一个事件监听器,用于处理 iframe 加载完成的事件。
4. 在 Vue 组件的方法中,处理 iframe 加载完成的事件:
```javascript
methods: {
handleIframeLoad() {
console.log('Iframe loaded');
// 在此处可以执行一些操作,比如向 iframe 发送消息等
}
}
```
通过使用 vue-iframe 组件,你可以更方便地在Vue应用中嵌入和操作iframe,而无需手动处理跨域等问题。你可以根据需要进一步探索 vue-iframe 的文档,了解更多关于它的用法和功能。
相关推荐
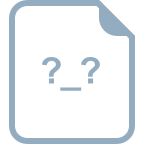
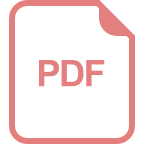
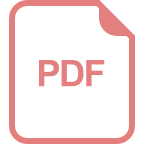












