代码生成(js读取本地txt)
时间: 2023-03-15 11:28:51 浏览: 77
我可以为你提供一个简单的示例:使用JavaScript读取本地txt文件的代码:<script type="text/javascript">
var reader = new FileReader();
reader.onload = function(e) {
var contents = e.target.result;
console.log(contents);
};
reader.readAsText(file);
</script>
相关问题
生成js读取本地文件夹并在页面显示所有图片的代码
以下是一个简单的实现,使用了HTML5的File API读取本地文件夹,并使用JavaScript动态创建img元素来显示所有图片:
```html
<!DOCTYPE html>
<html>
<head>
<title>Show Local Images</title>
<style>
#images {
display: flex;
flex-wrap: wrap;
justify-content: center;
}
img {
max-width: 200px;
max-height: 200px;
margin: 10px;
}
</style>
</head>
<body>
<input type="file" id="folderInput" webkitdirectory>
<div id="images"></div>
<script>
const folderInput = document.getElementById('folderInput');
const imagesDiv = document.getElementById('images');
folderInput.addEventListener('change', function(e) {
const files = e.target.files;
for (let i = 0; i < files.length; i++) {
if (files[i].type.match(/image.*/)) {
const reader = new FileReader();
reader.onload = function(e) {
const img = document.createElement('img');
img.src = e.target.result;
imagesDiv.appendChild(img);
};
reader.readAsDataURL(files[i]);
}
}
});
</script>
</body>
</html>
```
注意,这个代码需要在一个本地服务器上运行(例如使用VS Code中的Live Server插件)才能正常加载本地文件。
js实现读取本地目录生成文件树
实现读取本地目录生成文件树的方法有很多种,其中之一是使用递归函数遍历目录并生成树形结构。
以下是一个简单的示例代码:
```javascript
function generateTree(path) {
const fs = require('fs');
const stats = fs.statSync(path);
if (!stats.isDirectory()) {
// 如果不是目录则返回空
return null;
}
const tree = {
name: path.substring(path.lastIndexOf('/') + 1),
children: []
};
const files = fs.readdirSync(path);
files.forEach(file => {
const subPath = `${path}/${file}`;
const subTree = generateTree(subPath);
if (subTree) {
tree.children.push(subTree);
}
});
return tree;
}
// 调用示例
const tree = generateTree('/path/to/folder');
console.log(tree);
```
这段代码使用了 `fs` 模块来读取本地文件系统中的目录和文件信息,通过递归遍历来生成文件树结构。需要注意的是,这里只考虑了 Unix 风格的路径格式,如果在 Windows 环境下使用,需要修改路径分隔符。
相关推荐
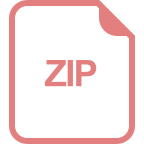
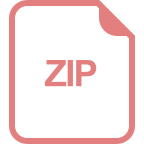












