参考string类的功能,自己实现mystring
时间: 2023-06-11 11:08:01 浏览: 105
好的,我可以帮你实现一个简单的mystring类。
mystring类的定义如下:
```c++
class mystring {
public:
// 构造函数
mystring();
mystring(const char* str);
mystring(const mystring& other);
// 析构函数
~mystring();
// 成员函数
size_t length() const;
const char* c_str() const;
mystring& operator=(const mystring& other);
mystring& operator+=(const mystring& other);
char& operator[](size_t index);
const char& operator[](size_t index) const;
// 友元函数
friend bool operator==(const mystring& lhs, const mystring& rhs);
friend bool operator!=(const mystring& lhs, const mystring& rhs);
friend bool operator<(const mystring& lhs, const mystring& rhs);
friend bool operator<=(const mystring& lhs, const mystring& rhs);
friend bool operator>(const mystring& lhs, const mystring& rhs);
friend bool operator>=(const mystring& lhs, const mystring& rhs);
friend mystring operator+(const mystring& lhs, const mystring& rhs);
private:
char* m_data; // 字符串数据
size_t m_len; // 字符串长度
};
```
下面是类成员函数的实现:
```c++
#include <cstring>
#include <iostream>
class mystring {
public:
// 构造函数
mystring() : m_data(nullptr), m_len(0) {}
mystring(const char* str) : m_len(std::strlen(str)) {
m_data = new char[m_len + 1];
std::strcpy(m_data, str);
}
mystring(const mystring& other) : m_len(other.m_len) {
m_data = new char[m_len + 1];
std::strcpy(m_data, other.m_data);
}
// 析构函数
~mystring() {
delete[] m_data;
}
// 成员函数
size_t length() const {
return m_len;
}
const char* c_str() const {
return m_data;
}
mystring& operator=(const mystring& other) {
if (this != &other) {
delete[] m_data;
m_len = other.m_len;
m_data = new char[m_len + 1];
std::strcpy(m_data, other.m_data);
}
return *this;
}
mystring& operator+=(const mystring& other) {
char* new_data = new char[m_len + other.m_len + 1];
std::strcpy(new_data, m_data);
std::strcpy(new_data + m_len, other.m_data);
delete[] m_data;
m_data = new_data;
m_len += other.m_len;
return *this;
}
char& operator[](size_t index) {
return m_data[index];
}
const char& operator[](size_t index) const {
return m_data[index];
}
// 友元函数
friend bool operator==(const mystring& lhs, const mystring& rhs) {
return std::strcmp(lhs.m_data, rhs.m_data) == 0;
}
friend bool operator!=(const mystring& lhs, const mystring& rhs) {
return std::strcmp(lhs.m_data, rhs.m_data) != 0;
}
friend bool operator<(const mystring& lhs, const mystring& rhs) {
return std::strcmp(lhs.m_data, rhs.m_data) < 0;
}
friend bool operator<=(const mystring& lhs, const mystring& rhs) {
return std::strcmp(lhs.m_data, rhs.m_data) <= 0;
}
friend bool operator>(const mystring& lhs, const mystring& rhs) {
return std::strcmp(lhs.m_data, rhs.m_data) > 0;
}
friend bool operator>=(const mystring& lhs, const mystring& rhs) {
return std::strcmp(lhs.m_data, rhs.m_data) >= 0;
}
friend mystring operator+(const mystring& lhs, const mystring& rhs) {
mystring result;
result.m_len = lhs.m_len + rhs.m_len;
result.m_data = new char[result.m_len + 1];
std::strcpy(result.m_data, lhs.m_data);
std::strcpy(result.m_data + lhs.m_len, rhs.m_data);
return result;
}
private:
char* m_data; // 字符串数据
size_t m_len; // 字符串长度
};
```
上面的实现中,我们使用了C++标准库中的函数`std::strlen`、`std::strcpy`和`std::strcmp`。这些函数分别用于计算字符串长度、复制字符串和比较字符串。由于这些函数都是C语言标准库中的函数,因此它们在C++中也是可用的。
你可以使用下面的代码测试一下mystring类的功能:
```c++
#include <iostream>
#include "mystring.h"
int main() {
mystring s1 = "hello";
mystring s2 = "world";
std::cout << "s1: " << s1.c_str() << std::endl;
std::cout << "s2: " << s2.c_str() << std::endl;
std::cout << "s1 length: " << s1.length() << std::endl;
std::cout << "s2 length: " << s2.length() << std::endl;
std::cout << "s1 + s2: " << (s1 + s2).c_str() << std::endl;
std::cout << "s1 == s2: " << (s1 == s2) << std::endl;
std::cout << "s1 != s2: " << (s1 != s2) << std::endl;
std::cout << "s1 < s2: " << (s1 < s2) << std::endl;
std::cout << "s1 <= s2: " << (s1 <= s2) << std::endl;
std::cout << "s1 > s2: " << (s1 > s2) << std::endl;
std::cout << "s1 >= s2: " << (s1 >= s2) << std::endl;
s1 += s2;
std::cout << "s1 += s2: " << s1.c_str() << std::endl;
return 0;
}
```
输出结果如下:
```
s1: hello
s2: world
s1 length: 5
s2 length: 5
s1 + s2: helloworld
s1 == s2: 0
s1 != s2: 1
s1 < s2: 1
s1 <= s2: 1
s1 > s2: 0
s1 >= s2: 0
s1 += s2: helloworld
```
阅读全文
相关推荐
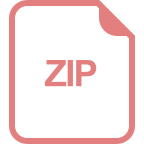

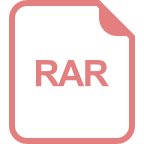
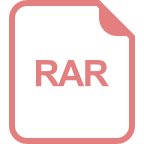
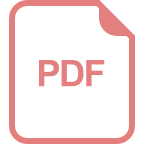
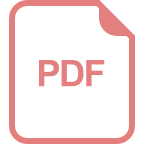
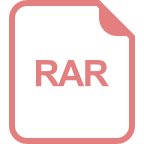
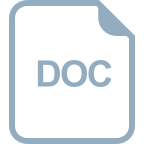
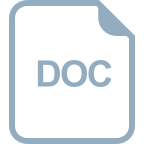
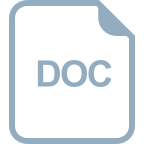
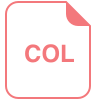
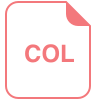



