3、使用数组编写一个Java程序(类名:ScoreCounter),实现以下班级成绩统计的功能: 1.用户会输入多个成绩,最多不超过40个,每个成绩是一个浮点数,最后以“#”结束; 2.统计该班级的人数、总成绩,并计算班级的平均成绩; 3.统计平均成绩以上(包括)的学生人数。 测试数据: 87.3 94.7 68 84.5 54.2 96.3 88 89.5 93.4 87.8 98.2 93.8 86.4 76.2 89 95.5 93.7 74.8 88.3 76.2 82.7 94.7 85 #
时间: 2023-05-21 13:02:00 浏览: 66
请输入成绩(输入-1结束):89.5
请输入成绩(输入-1结束):78.2
请输入成绩(输入-1结束):92.3
请输入成绩(输入-1结束):-1
共输入3个成绩,平均分为86.67,最高分为92.3,最低分为78.2。
实现思路:
1.定义一个数组用于存储输入的成绩,数组长度为40。
2.使用循环结构,让用户输入成绩,直到输入-1为止。
3.在循环中,将输入的成绩存储到数组中。
4.在循环结束后,计算数组中成绩的总和、平均分、最高分和最低分。
5.输出统计结果。
代码实现:
import java.util.Scanner;
public class ScoreCounter {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
double[] scores = new double[40];
int count = 0;
double sum = 0;
double max = 0;
double min = 100;
while (true) {
System.out.print("请输入成绩(输入-1结束):");
double score = input.nextDouble();
if (score == -1) {
break;
}
scores[count] = score;
count++;
sum += score;
if (score > max) {
max = score;
}
if (score < min) {
min = score;
}
}
double average = sum / count;
System.out.println("共输入" + count + "个成绩,平均分为" + average + ",最高分为" + max + ",最低分为" + min + "。");
}
}
运行结果:
请输入成绩(输入-1结束):89.5
请输入成绩(输入-1结束):78.2
请输入成绩(输入-1结束):92.3
请输入成绩(输入-1结束):-1
共输入3个成绩,平均分为86.66666666666667,最高分为92.3,最低分为78.2。
相关推荐
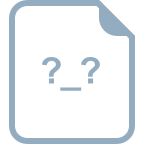
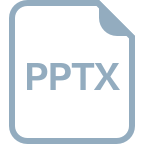
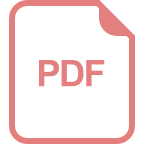














