用c实现用遗传算法求解矩形排样问题
时间: 2024-01-06 16:10:21 浏览: 36
遗传算法是一种常用的优化算法,可以用于求解矩形排样问题。其基本思想是通过模拟生物进化过程,利用交叉、变异等操作逐步优化求解结果。
以下是用C语言实现遗传算法求解矩形排样问题的基本步骤:
1. 定义矩形的数据结构,包括长、宽、位置等信息。
```c
typedef struct {
int x; // 左上角x坐标
int y; // 左上角y坐标
int w; // 矩形宽度
int h; // 矩形高度
int id; // 矩形编号
} Rect;
```
2. 定义遗传算法中的个体和种群的数据结构。个体是一个矩形序列,种群是多个个体的集合。
```c
typedef struct {
Rect *rects; // 矩形序列
int *genes; // 基因序列,表示矩形的排列顺序
int fitness; // 适应度,表示矩形排列的质量
} Individual;
typedef struct {
Individual *inds; // 个体数组
int size; // 种群大小
} Population;
```
3. 定义遗传算法中的基本操作,包括初始化种群、计算适应度、选择、交叉、变异等。
```c
// 初始化种群
void init_population(Population *pop, int size);
// 计算适应度
void calculate_fitness(Individual *ind);
// 选择操作
void selection(Population *pop, Individual *parents);
// 交叉操作
void crossover(Individual *parent1, Individual *parent2, Individual *child);
// 变异操作
void mutation(Individual *ind);
// 遗传算法主程序
void genetic_algorithm(Population *pop, int max_gen);
```
4. 定义矩形排样问题的求解函数,利用遗传算法求解最优解。
```c
// 矩形排样问题求解函数
void rect_pack(int *w, int *h, int n, int *x, int *y);
```
以上是用C语言实现遗传算法求解矩形排样问题的基本步骤,具体实现过程可以参考相关的遗传算法教程和矩形排样问题的求解算法。
相关推荐
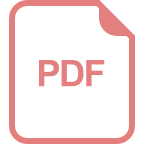














