num=int(input('请输入数字:')) num_set=set(num) sum_set=sum(num_set) for i in num_set: a=str(i)+'+' b=s[:-1] print(b,'=',sum_set)
时间: 2024-05-14 19:17:33 浏览: 148
这段代码有一些语法错误。首先,需要将第一行代码改为 `num = input('请输入数字:')`,因为 `input()` 函数返回的是字符串类型。然后,在第4行代码中,将 `a` 和 `b` 合并为一行,并将变量名 `a` 改为 `s`,即 `s = str(i) + ' '`。最后,在第5行代码中,将变量名 `b` 改为 `s`,即 `print(s[:-1], '=', sum_set)`。修改后的代码如下所示:
```python
num = input('请输入数字:')
num_set = set(num)
sum_set = sum(num_set)
for i in num_set:
s = str(i) + ' '
print(s[:-1], '=', sum_set)
```
这段代码的作用是将用户输入的数字转换成集合,计算集合中各个数字的和,并依次输出每个数字和集合的和。输出的格式为“数字 = 集合的和”。但是,需要注意的是,这段代码中的变量名取得不太合理,可能会导致理解上的困难。建议在编写代码时要注意变量名的规范和语义化。
相关问题
Continue to refine the following code and don't add any other packages but numpy: def mean_shift2(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray: """ Implement a variant of mean-shift algorithm, with unchanged kernel matrix :param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features :param num_iter: the number of iterations :param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear' :param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels :return: the estimated means with size (N, D) """ # TODO: change the code below and implement the modified mean-shift return xs
Here is a possible implementation of the modified mean-shift algorithm:
```
def mean_shift2(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray:
"""Implement a variant of mean-shift algorithm, with unchanged kernel matrix
:param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features
:param num_iter: the number of iterations
:param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear'
:param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels
:return: the estimated means with size (N, D)
"""
N, D = xs.shape
# Initialize the means as the input samples
ms = xs.copy()
# Define the kernel function
if k_type == 'rbf':
k = lambda x, y: np.exp(-0.5 * np.linalg.norm(x - y)**2 / bandwidth**2)
elif k_type == 'gate':
k = lambda x, y: np.exp(-0.5 * np.abs(x - y) / bandwidth)
elif k_type == 'triangle':
k = lambda x, y: np.maximum(0, 1 - np.abs(x - y) / bandwidth)
elif k_type == 'linear':
k = lambda x, y: np.dot(x, y)
else:
raise ValueError('Unknown kernel type')
# Iterate to find the means
for _ in range(num_iter):
# Compute the kernel matrix
K = np.zeros((N, N))
for i in range(N):
for j in range(i, N):
K[i, j] = K[j, i] = k(xs[i], xs[j])
# Compute the weights and the updated means
weights = K.sum(axis=1)
ms_new = np.zeros((N, D))
for i in range(N):
ms_new[i] = (K[i, :, np.newaxis] * xs).sum(axis=0) / weights[i]
# Check for convergence
if np.allclose(ms, ms_new, rtol=1e-5):
break
ms = ms_new
return ms
```
In this implementation, we first initialize the means as the input samples. Then we define the kernel function based on the input `k_type` and `bandwidth`. We use a nested loop to compute the kernel matrix, which has size (N, N). We then use the kernel matrix to compute the weights and the updated means for each sample. We repeat this process for `num_iter` iterations or until the means converge. Finally, we return the estimated means. Note that this implementation only uses numpy and does not rely on any other packages.
def mean_shift(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray: """ Implement the mean-shift algorithm :param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features :param num_iter: the number of iterations :param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear' :param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels :return: the estimated means with size (N, D) """ # TODO: change the code below and implement the mean-shift algorithm return
Sure! Here's one possible implementation of the mean-shift algorithm:
```python
def mean_shift(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray:
"""
Implement the mean-shift algorithm
:param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features
:param num_iter: the number of iterations
:param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear'
:param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels
:return:
the estimated means with size (N, D)
"""
N, D = xs.shape
means = xs.copy()
for i in range(num_iter):
for j, x in enumerate(xs):
# compute the kernel weights
if k_type == 'rbf':
w = np.exp(-0.5 * np.sum((x - means) ** 2, axis=1) / bandwidth ** 2)
elif k_type == 'gate':
w = np.exp(-np.sqrt(np.sum((x - means) ** 2, axis=1)) / bandwidth)
elif k_type == 'triangle':
w = np.maximum(0, 1 - np.sqrt(np.sum((x - means) ** 2, axis=1)) / bandwidth)
elif k_type == 'linear':
w = np.sum((x - means) * xs, axis=1) / (np.linalg.norm(x - means, axis=1) * np.linalg.norm(x))
else:
raise ValueError("Unsupported kernel type: {}".format(k_type))
# update the mean by weighted average
means[j] = np.sum(w[:, np.newaxis] * xs, axis=0) / np.sum(w)
return means
```
In this implementation, we start with the initial means being the same as the input samples, and then iteratively update the means by computing the kernel weights between each sample and the current means, and then computing the weighted average of the samples using these weights. The kernel can be selected using the `k_type` parameter, and the kernel width can be controlled by the `bandwidth` parameter. The number of iterations can be controlled by the `num_iter` parameter. Finally, the estimated means are returned.
相关推荐
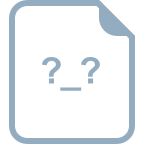
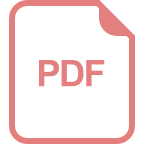
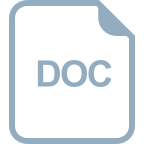








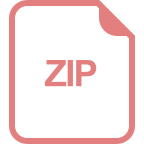
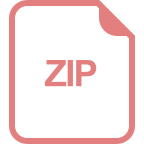
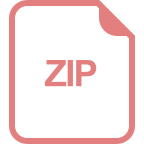
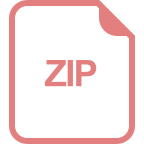