Consider two 4-bit binary numbers A and B. Bits of A are a3, a2, a1, and a0, and bits of B are b3, b2, b1, and b0. A is greater than B if a3 is 1 and b3 is 0, but if a3 and b3 are the same, then if a2 is 1 and b2 is 0, we can determine that A is greater than B. This evaluation continues until a0 and b0 are considered. If a0 and b0 are equal then A and B are equal. Using discrete gates and Verilog gate primitives build a 4-bit comparator that generates a 1 on its GT output when its 4-bit input A is greater than its B input, and a 1 on its EQ output when A and B are equal. Use the generate statement and other Verilog iterative logic support structures.
时间: 2023-12-22 20:02:52 浏览: 24
Here is one possible implementation of a 4-bit comparator in Verilog:
```verilog
module comparator (
input [3:0] A,
input [3:0] B,
output GT,
output EQ
);
// Generate the comparison logic for each bit position
genvar i;
generate
for (i = 3; i >= 0; i--) begin
assign GT = (A[i] > B[i]) ? 1 : 0;
assign EQ = (A[i] == B[i]) ? 1 : 0;
if (i > 0) begin
assign GT = (A[i] == B[i]) ? GT : (!A[i] & B[i]);
end
end
endgenerate
endmodule
```
This implementation uses a `generate` block to create the comparison logic for each bit position. The first two `assign` statements check if the current bit of `A` is greater than or equal to the current bit of `B`, and set `GT` and `EQ` accordingly. The third `assign` statement checks if the current bits of `A` and `B` are equal, and if they are, it keeps the previous value of `GT`, since the current bit doesn't affect the comparison. If the current bits of `A` and `B` are not equal, then `GT` is set to 1 if `A` has a 1 in that position and `B` has a 0, otherwise it stays the same.
Note that this implementation assumes that `A` and `B` are always 4-bit inputs, and does not handle cases where they are different sizes. It also does not handle signed or two's complement numbers.
相关推荐
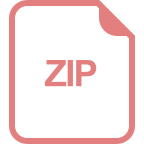
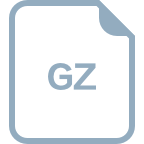
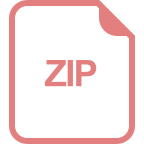
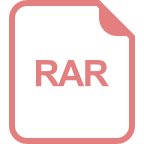
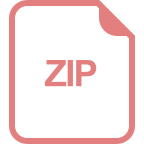
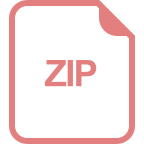
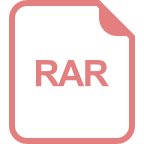
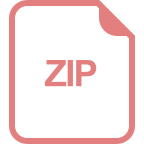
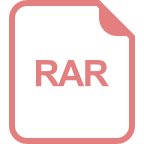
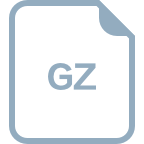
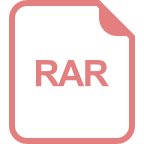
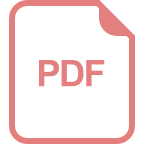
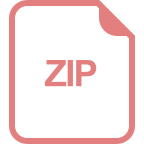