python怎么用numpy将自定义类型输出写入txt
时间: 2024-10-24 22:06:04 浏览: 49
在Python中,如果你想要使用NumPy将自定义类型的数组写入文本文件(txt),你可以这样做:
首先,确保你的自定义类型有一个`__repr__`方法,这个方法返回字符串形式的表示,因为NumPy需要能够将其转换为字符串。例如,如果你有一个名为`CustomType`的简单自定义类,你可以添加如下`__repr__`方法:
```python
class CustomType:
def __init__(self, value):
self.value = value
def __repr__(self):
return f"CustomType({self.value})"
```
接下来,你可以创建一个包含这种类型的NumPy数组,并使用`numpy.savetxt`函数来保存它到txt文件:
```python
import numpy as np
# 创建一个示例的CustomType数组
custom_array = [CustomType(i) for i in range(5)]
# 将CustomType数组转换为普通的Python列表(如果可能的话)
# 因为numpy.savetxt需要基本的数据类型
array_to_save = [item.value for item in custom_array]
# 写入txt文件
np.savetxt('output.txt', array_to_save, fmt='%s')
# 如果你想保留原始结构并使用NumPy的复杂数据类型支持,可以考虑使用numpy.savez_compressed或pickle模块
# 但是通常建议先转化为基础数据类型再保存,以保持兼容性和易于读取
# np.savez_compressed('output.npz', data=custom_array)
# 或者
# with open('output.pkl', 'wb') as f:
# pickle.dump(custom_array, f)
```
阅读全文
相关推荐














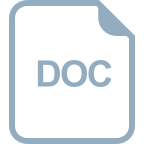



