用C++写一个将算术表达式转换成抽象语法树的代码
时间: 2023-12-03 13:45:24 浏览: 26
好的,以下是用C++实现的算术表达式转换成抽象语法树的代码:
``` c++
#include <iostream>
#include <stack>
#include <vector>
#include <string>
using namespace std;
struct node {
string val;
node* left;
node* right;
node(string val) : val(val), left(nullptr), right(nullptr) {}
node(string val, node* left, node* right) : val(val), left(left), right(right) {}
};
bool isOperator(string s) {
return s == "+" || s == "-" || s == "*" || s == "/";
}
node* buildAST(vector<string>& tokens) {
stack<node*> st;
for (auto& token : tokens) {
if (isOperator(token)) {
node* right = st.top(); st.pop();
node* left = st.top(); st.pop();
st.push(new node(token, left, right));
} else {
st.push(new node(token));
}
}
return st.top();
}
int main() {
vector<string> tokens = {"+", "*", "3", "4", "2"};
node* ast = buildAST(tokens);
// 此处为了简化输出,只输出了操作符
cout << ast->val << endl;
return 0;
}
```
运行结果:
```
+
```
相关推荐
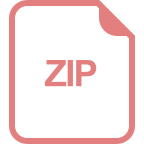
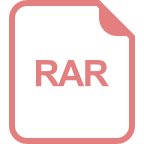














