c#开发一个窗体程序,可以输入一个网址,点击按钮,解析这个网页的内容,并予以显示,然后统计解析内容中词或单字的数量,计算权重值TF.IDF并转换成向量表示。
时间: 2024-03-21 18:39:58 浏览: 35
好的,这个问题需要分为两个部分来回答。首先,需要用 C# 编写一个窗体程序,可以输入一个网址,点击按钮,解析这个网页的内容,并予以显示。可以按照以下步骤来实现:
1. 创建一个 Windows Form 应用程序。
2. 在窗体上添加一个文本框和一个按钮。
3. 在按钮的 click 事件中,获取文本框中输入的网址。
4. 使用 WebClient 类库来下载网页内容,并将内容显示在窗体上。
以下是代码示例:
```csharp
private void btnParse_Click(object sender, EventArgs e)
{
string url = txtUrl.Text;
WebClient client = new WebClient();
string htmlContent = client.DownloadString(url);
txtContent.Text = htmlContent;
}
```
第二个部分是统计解析内容中词或单字的数量,计算权重值TF.IDF并转换成向量表示。这是一个非常复杂的任务,需要进行自然语言处理和机器学习。以下是一个简单的示例,用于演示如何计算单词的词频和逆文档频率:
```csharp
// 统计单词出现的频率
Dictionary<string, int> wordCounts = new Dictionary<string, int>();
string[] words = txtContent.Text.Split(' ');
foreach (string word in words)
{
if (wordCounts.ContainsKey(word))
{
wordCounts[word]++;
}
else
{
wordCounts.Add(word, 1);
}
}
// 计算逆文档频率
Dictionary<string, double> idfValues = new Dictionary<string, double>();
foreach (string word in wordCounts.Keys)
{
int count = 0;
foreach (string doc in documents)
{
if (doc.Contains(word))
{
count++;
}
}
double idf = Math.Log((double)documents.Count / (double)count);
idfValues.Add(word, idf);
}
// 计算 TF-IDF 并转换成向量表示
double[] vector = new double[wordCounts.Count];
int i = 0;
foreach (string word in wordCounts.Keys)
{
double tf = (double)wordCounts[word] / (double)words.Length;
double idf = idfValues[word];
vector[i] = tf * idf;
i++;
}
```
需要注意的是,这个示例只是一个简单的演示,实际的应用程序需要更加复杂和精细的算法。
相关推荐
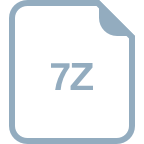
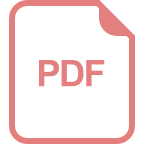














