如何随机取一个集合中的元素
时间: 2024-09-24 10:29:08 浏览: 31
在Python中,如果你有一个列表(list)、元组(tuple)或集合(set),你可以使用`random.choice()`函数来随机选择一个元素。这个函数是Python标准库`random`模块的一部分。
```python
import random
# 假设你有一个列表
my_list = ['apple', 'banana', 'cherry', 'date']
# 随机选取一个元素
random_element = random.choice(my_list)
print(random_element)
```
对于集合(set),虽然它们不支持索引直接访问元素,但也可以通过转换为列表再进行随机选择:
```python
my_set = {'orange', 'pear', 'grape'}
# 先转为列表
my_list_from_set = list(my_set)
random_element_from_set = random.choice(my_list_from_set)
print(random_element_from_set)
```
如果你想从集合中保持其无重复的特点,确保你在选择后再次将其转换回集合,否则可能会失去这个特性:
```python
random_element_from_set = random.choice(list(my_set))
random_set = {random_element_from_set} # 注意这里创建了一个新的集合
```
相关问题
java set集合随机取元素_Java集合系列-Set系列-HashSet
可以使用Java中的Random类配合Set的toArray方法来随机获取Set集合中的元素,具体代码如下:
```java
import java.util.HashSet;
import java.util.Random;
import java.util.Set;
public class RandomSetElement {
public static void main(String[] args) {
Set<String> set = new HashSet<>();
set.add("A");
set.add("B");
set.add("C");
set.add("D");
set.add("E");
String[] array = set.toArray(new String[set.size()]);
Random random = new Random();
int index = random.nextInt(array.length);
String randomElement = array[index];
System.out.println("随机获取的元素为:" + randomElement);
}
}
```
首先将Set集合转换为数组,然后使用Random类生成一个随机数,最后通过数组下标获取随机元素。
请使用python编写一个访问控制模型的程序,实现以下功能:1.创建主体S={sa1,sa2,...,sa10}、客体O={oa1,oa2,...,oa10}、环境E={ea1,ea2,...,ea10}以及操作OP={}。生成策略p=<SR,OR,ER,op,effect>,其中SR是主体S中3到5个元素间随机的bool组合的集合,组合数量为3到5个;OR是客体O中3到5个元素间随机的bool组合的集合,组合数量为3到5个;ER是环境E中3到5个元素间随机的bool组合的集合,组合数量为3到5个;op是集合{op1,op2,op3,op4};effect属于集合{permit,deny}。 2.程序中包含可信引用监控器,接收用户的访问请求,请求内容为四个元素的集合,前三个元素是bool组合,请在SA、OA、EA中分别核查是否有第一个元素、第二个元素、第三个元素的存在,在op中核查是否有第四个元素的存在。如果核查中有元素不在集合中存在或者冲突时effect取为deny,否则取为permit。 3.请随机生成5000个正决策p1,5000个负决策p0,随机生成10000个请求,进行决策,将决策结果记录到第一个文本数据库中,记录主体S、客体O、环境E、操作OP到第二个文本数据库中,记录生成的请求到第三个文本数据库中。
以下是一个实现以上功能的示例代码:
```python
import random
class Subject:
def __init__(self, name, attributes):
self.name = name
self.attributes = attributes
class Object:
def __init__(self, name, attributes):
self.name = name
self.attributes = attributes
class Environment:
def __init__(self, name, attributes):
self.name = name
self.attributes = attributes
class Policy:
def __init__(self, subject_set, object_set, environment_set, operation_set, effect):
self.subject_set = subject_set
self.object_set = object_set
self.environment_set = environment_set
self.operation_set = operation_set
self.effect = effect
class AccessRequest:
def __init__(self, subject_attributes, object_attributes, environment_attributes, operation):
self.subject_attributes = subject_attributes
self.object_attributes = object_attributes
self.environment_attributes = environment_attributes
self.operation = operation
class AccessControl:
def __init__(self, policies):
self.policies = policies
def check_access(self, access_request):
for policy in self.policies:
if all(attr in policy.subject_set or not attr for attr in access_request.subject_attributes) \
and all(attr in policy.object_set or not attr for attr in access_request.object_attributes) \
and all(attr in policy.environment_set or not attr for attr in access_request.environment_attributes) \
and access_request.operation in policy.operation_set:
return policy.effect
return 'deny'
# 生成主体、客体、环境
s_list = [Subject(f'sa{i}', [random.choice([True, False]) for _ in range(random.randint(3, 5))]) for i in range(1, 11)]
o_list = [Object(f'oa{i}', [random.choice([True, False]) for _ in range(random.randint(3, 5))]) for i in range(1, 11)]
e_list = [Environment(f'ea{i}', [random.choice([True, False]) for _ in range(random.randint(3, 5))]) for i in range(1, 11)]
# 生成策略
policies = []
for i in range(10000):
subject_set = set(random.sample(s_list, random.randint(3, 5)))
object_set = set(random.sample(o_list, random.randint(3, 5)))
environment_set = set(random.sample(e_list, random.randint(3, 5)))
operation_set = set(random.sample(['op1', 'op2', 'op3', 'op4'], random.randint(1, 4)))
effect = random.choice(['permit', 'deny'])
policies.append(Policy(subject_set, object_set, environment_set, operation_set, effect))
# 记录策略
with open('policy_db.txt', 'w') as f:
for policy in policies:
s_str = ','.join([s.name for s in policy.subject_set])
o_str = ','.join([o.name for o in policy.object_set])
e_str = ','.join([e.name for e in policy.environment_set])
op_str = ','.join(policy.operation_set)
f.write(f'{s_str} / {o_str} / {e_str} / {op_str} / {policy.effect}\n')
# 生成请求
requests = []
for i in range(10000):
subject_attributes = [random.choice([True, False]) for _ in range(random.randint(1, 3))]
object_attributes = [random.choice([True, False]) for _ in range(random.randint(1, 3))]
environment_attributes = [random.choice([True, False]) for _ in range(random.randint(1, 3))]
operation = random.choice(['op1', 'op2', 'op3', 'op4'])
requests.append(AccessRequest(subject_attributes, object_attributes, environment_attributes, operation))
# 记录请求
with open('request_db.txt', 'w') as f:
for request in requests:
s_str = ','.join([str(attr) for attr in request.subject_attributes])
o_str = ','.join([str(attr) for attr in request.object_attributes])
e_str = ','.join([str(attr) for attr in request.environment_attributes])
f.write(f'{s_str} / {o_str} / {e_str} / {request.operation}\n')
# 进行决策
access_control = AccessControl(policies)
results = []
for request in requests:
result = access_control.check_access(request)
results.append(result)
# 记录决策结果
with open('result_db.txt', 'w') as f:
for result in results:
f.write(f'{result}\n')
```
以上示例中,我们首先生成了主体、客体、环境等基本元素。然后,随机生成了一定数量的策略和请求。通过 AccessControl 类实现对请求进行访问控制,返回访问结果。最后,将策略、请求和访问结果分别记录到不同的文本数据库中。
阅读全文
相关推荐
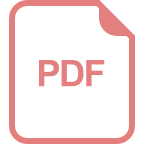
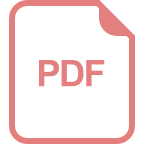
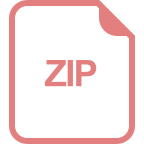
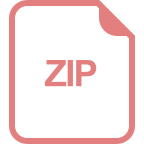
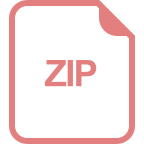
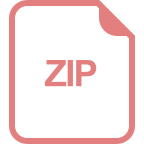










