while True : with open('/home/pi/Documents/8-17/simplest_output.txt', 'w') as f: proc = subprocess.Popen(["python3", "/home/pi/Documents/8-17/simplest.py"], stdout=f, stderr=f) proc.wait() # 等待子进程执行完毕 if proc.returncode == 0: print("simplest运行正常.....") break 主窗口依然不会打印simplest运行正常
时间: 2023-12-10 12:38:53 浏览: 34
这是因为你在子进程中将输出重定向到了文件 `/home/pi/Documents/8-17/simplest_output.txt`,而没有将输出传递回父进程。你可以在子进程中打印一些信息,以便在主窗口中查看。
例如,在子进程中添加以下代码:
```python
print("simplest运行正常.....")
```
这样当子进程正常退出时,就会在 `/home/pi/Documents/8-17/simplest_output.txt` 文件中输出该信息,同时也会在主窗口中打印出来。
相关问题
while True: proc = subprocess.Popen(["python3", "/home/pi/Documents/8-17/simplest.py"]) while proc.poll() is None: time.sleep(1) print("simplest运行中.....") chinanet = isNetChainOK() if chinanet is False: print("网络异常") for proc in psutil.process_iter(): if proc.name() == 'simplest.py': print("simplest受网络问题强行中断") proc.terminate() # or proc.kill() proc = subprocess.Popen(["python3", "/home/pi/Documents/8-17/simplest.py"]) getNet() 断网后报错:while proc.poll() is None: AttributeError: 'Process' object has no attribute 'poll'
这个错误可能是因为 subprocess.Popen() 方法返回的对象类型不是 Process 类型,而是 NoneType 类型。这可能是由于 subprocess.Popen() 调用失败导致的。
你可以在调用 subprocess.Popen() 方法之前添加一些错误处理代码,以避免此错误。例如:
```
while True:
try:
proc = subprocess.Popen(["python3", "/home/pi/Documents/8-17/simplest.py"])
break
except Exception as e:
print("Error starting process:", e)
time.sleep(1)
while proc.poll() is None:
time.sleep(1)
print("simplest运行中.....")
chinanet = isNetChainOK()
if chinanet is False:
print("网络异常")
for proc in psutil.process_iter():
if proc.name() == 'simplest.py':
print("simplest受网络问题强行中断")
proc.terminate() # or proc.kill()
proc = subprocess.Popen(["python3", "/home/pi/Documents/8-17/simplest.py"])
getNet()
```
这样,如果 subprocess.Popen() 调用失败,代码将等待 1 秒钟,然后重试。如果调用成功,代码将继续执行。
import subprocess while True: proc = subprocess.Popen(["python3", "/home/pi/Documents/8-17/simplest.py"]) try: while True: if proc.poll() is not None: break print("simplest运行成功.....") except KeyboardInterrupt: proc.terminate() break 这样simplest死循环里打印的信息不会出现在终端
这是因为 `subprocess.Popen` 创建的子进程是在独立的进程空间内执行的,并不会把输出打印到终端。要想在终端打印子进程的输出,可以使用 `subprocess.PIPE` 来捕获子进程的标准输出和标准错误输出,然后在父进程中打印出来。修改代码如下:
```python
import subprocess
while True:
proc = subprocess.Popen(["python3", "/home/pi/Documents/8-17/simplest.py"],
stdout=subprocess.PIPE, stderr=subprocess.PIPE)
try:
while True:
if proc.poll() is not None:
break
# 读取子进程的输出
output = proc.stdout.readline().decode().strip()
if output:
print(output)
error = proc.stderr.readline().decode().strip()
if error:
print(error)
except KeyboardInterrupt:
proc.terminate()
break
```
这样就可以实时打印子进程的输出了。
阅读全文
相关推荐
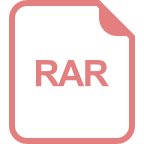
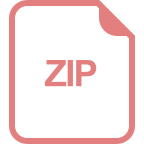
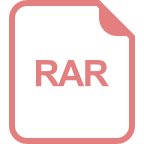


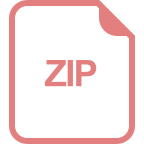
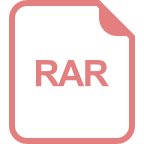
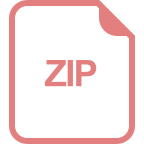
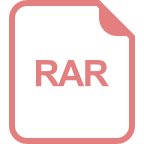
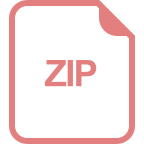
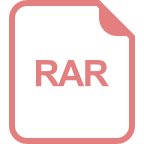
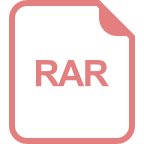
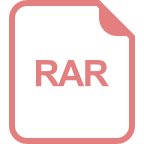
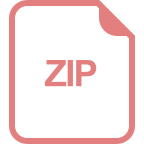
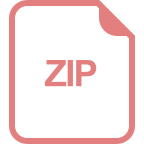