输人若干个学生信息(包括学号、姓名和成绩),输人学号为0时输人结束,建立一个单向链表,再输人一个成绩值,将成绩大于等于该值的学生信息输出。试编写相应程序。
时间: 2023-06-01 19:01:44 浏览: 82
### 回答1:
好的,以下是程序的中文描述:
1. 定义一个结构体,包含学号、姓名和成绩三个成员变量。
2. 定义一个单向链表结构体,包含一个指向头节点的指针。
3. 通过循环输入学生信息,当输入的学号为时结束循环。
4. 每输入一个学生信息,就新建一个节点,将学生信息存储在节点中,并将节点插入链表中。
5. 输入一个成绩值,遍历链表,将成绩大于等于该值的学生信息输出。
以下是程序的代码实现:
### 回答2:
这是一个简单的链表操作问题。
首先,我们需要定义链表节点的数据结构。因为每个节点都包含学号、姓名和成绩这三个信息,所以我们可以这样定义:
```
struct Student {
int id;
char name[20];
int score;
struct Student* next;
};
```
其中,id表示学号,name是姓名,score是成绩,next是指向下一个节点的指针。
接下来,我们可以编写一个函数来读入学生信息并创建链表:
```
struct Student* createList() {
struct Student* head = NULL;
struct Student* tail = NULL;
int id;
char name[20];
int score;
printf("请输入学生信息(学号 姓名 成绩):\n");
while (1) {
scanf("%d", &id);
if (id == 0) {
break;
}
scanf("%s %d", name, &score);
struct Student* node = (struct Student*)malloc(sizeof(struct Student));
node->id = id;
strcpy(node->name, name);
node->score = score;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
```
这个函数会不断读入学生信息,并创建节点加入链表中,直到输入学号为0为止。
接下来,我们编写一个函数来输出成绩大于等于给定值的学生信息:
```
void printAbove(struct Student* head, int score) {
struct Student* node = head;
int count = 0;
printf("成绩大于等于%d的学生信息:\n", score);
while (node != NULL) {
if (node->score >= score) {
printf("%d %s %d\n", node->id, node->name, node->score);
count++;
}
node = node->next;
}
if (count == 0) {
printf("没有成绩大于等于%d的学生\n", score);
}
}
```
这个函数接受一个链表头和一个分数值,遍历链表,输出成绩大于等于该值的学生信息。如果没有满足条件的学生,就输出一条提示信息。
最后,我们可以将两个函数结合起来,在main函数中编写代码,实现完整的程序:
```
int main() {
struct Student* head = createList();
int score;
printf("请输入成绩值:");
scanf("%d", &score);
printAbove(head, score);
return 0;
}
```
这个程序会先读入学生信息并创建链表,然后读入一个分数值,最后输出成绩大于等于该值的学生信息。
### 回答3:
为了实现这个功能,我们需要定义一个学生结构体,其中包含学号、姓名和成绩三个成员变量,如下所示:
```
struct Student {
int id;
char name[20];
double score;
Student *next;
};
```
接着,我们可以编写一个函数来输入学生信息并建立一个单向链表:
```
void inputStudents(Student *&head) {
head = nullptr;
Student *p, *q;
while (true) {
int id;
char name[20];
double score;
printf("请输入学号(输入0结束):");
scanf("%d", &id);
if (id == 0) {
break;
}
printf("请输入姓名:");
scanf("%s", name);
printf("请输入成绩:");
scanf("%lf", &score);
p = new Student;
p->id = id;
strcpy(p->name, name);
p->score = score;
p->next = nullptr;
if (head == nullptr) {
head = p;
} else {
q->next = p;
}
q = p;
}
}
```
注意,在这个函数中,我们需要使用两个指针变量p和q来建立链表,并在每次循环结束后将q指向p,以便下一次循环时将新节点连接到链表的末尾。
接下来,我们需要编写一个函数来输出所有成绩大于等于指定值的学生信息:
```
void outputStudents(Student *head, double threshold) {
printf("成绩大于等于%.2lf的学生有:\n", threshold);
for (Student *p = head; p != nullptr; p = p->next) {
if (p->score >= threshold) {
printf("%d\t%s\t%.2lf\n", p->id, p->name, p->score);
}
}
}
```
最后,我们只需要在主函数中依次调用这两个函数即可实现程序的功能:
```
int main() {
Student *head;
inputStudents(head);
double threshold;
printf("请输入成绩阈值:");
scanf("%lf", &threshold);
outputStudents(head, threshold);
return 0;
}
```
相关推荐
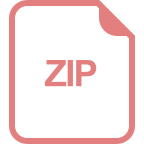
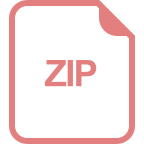














