p5908 猫猫和企鹅的代码
时间: 2024-08-17 08:01:42 浏览: 48
很抱歉,您提到的 "p5908 猫猫和企鹅的代码" 没有提供足够的上下文信息让我直接理解是指哪一段特定的代码。这通常可能是某个编程挑战、游戏项目或是某种编程语言(如Python、Java)中的示例代码,涉及到的角色是猫猫和企鹅。
一般来说,如果这是一个编程题目,它可能会涉及角色设计、用户交互或者游戏逻辑。例如,可能是一个简单的2D游戏,其中猫猫和企鹅作为游戏角色,各自有自己的移动、攻击等行为。
如果您能提供更多详细信息,比如这是关于哪种编程语言的,或者这个代码片段的具体功能,我会更乐意帮助解释或分析代码。同时,如果你正在寻找如何编写这样的代码,我可以指导你从基本的游戏框架开始,包括创建角色类,定义它们的行为方法。
相关问题
使用unity依赖,C#编写躲猫猫代码
以下是一个简单的躲猫猫游戏的示例代码,使用Unity的依赖和C#编写:
```
using UnityEngine;
public class HideAndSeek : MonoBehaviour
{
public GameObject cat;
public Transform[] hidingSpots;
private int currentHidingSpotIndex;
private bool isGameRunning;
private void Start()
{
currentHidingSpotIndex = 0;
isGameRunning = true;
cat.SetActive(false);
}
private void Update()
{
if (isGameRunning)
{
if (Input.GetKeyDown(KeyCode.Space))
{
StartGame();
}
else if (Input.GetKeyDown(KeyCode.UpArrow))
{
CheckHidingSpot();
}
}
}
private void StartGame()
{
cat.SetActive(true);
currentHidingSpotIndex = Random.Range(0, hidingSpots.Length);
}
private void CheckHidingSpot()
{
if (cat.activeSelf)
{
float distance = Vector3.Distance(cat.transform.position, hidingSpots[currentHidingSpotIndex].position);
if (distance < 2f)
{
Debug.Log("You win!");
isGameRunning = false;
}
else
{
Debug.Log("Try again!");
}
}
else
{
Debug.Log("Start the game first!");
}
}
}
```
这个示例中,我们有一个 `HideAndSeek` 类,它有三个公共变量:一个猫的 `GameObject`,一个隐藏点的 `Transform` 数组和一个当前隐藏点的索引。`Start` 方法初始化了游戏状态,将猫隐藏,并将当前隐藏点索引设置为0。`Update` 方法检查玩家的输入并调用对应的方法。如果玩家按下空格键,游戏开始,如果玩家按下向上箭头键,检查当前隐藏点是否正确。如果玩家赢了,游戏结束,如果玩家输了,继续尝试。
在 `StartGame` 方法中,我们将猫显示出来,并随机选择一个隐藏点。在 `CheckHidingSpot` 方法中,我们检查猫是否显示出来,如果是,我们计算猫和当前隐藏点之间的距离。如果距离小于2个单位,则玩家赢得了游戏,否则他们需要继续尝试。
使用unity依赖,C#编写直播互动游戏躲猫猫代码
以下是一个简单的躲猫猫游戏的代码示例,使用Unity和C#编写。这个游戏中,一个玩家是猫,其他玩家是老鼠。猫需要追逐老鼠,老鼠需要躲避猫,如果猫抓住了老鼠,老鼠就会变成猫。
```csharp
using UnityEngine;
using System.Collections.Generic;
public class HideAndSeekGame : MonoBehaviour {
public GameObject catPrefab;
public GameObject mousePrefab;
public Transform[] spawnPoints;
public float gameDuration = 60f;
public int miceToWin = 3;
private List<GameObject> mice = new List<GameObject>();
private GameObject cat;
private float gameTimer;
private int miceCaught;
void Start () {
// Spawn the cat
int catSpawnPoint = Random.Range(0, spawnPoints.Length);
cat = Instantiate(catPrefab, spawnPoints[catSpawnPoint].position, Quaternion.identity) as GameObject;
// Spawn the mice
for (int i = 0; i < spawnPoints.Length; i++) {
if (i != catSpawnPoint) {
GameObject mouse = Instantiate(mousePrefab, spawnPoints[i].position, Quaternion.identity) as GameObject;
mice.Add(mouse);
}
}
// Set game timer
gameTimer = gameDuration;
}
void Update () {
if (gameTimer > 0f) {
// Count down the game timer
gameTimer -= Time.deltaTime;
// Check if a mouse has been caught
foreach (GameObject mouse in mice) {
MouseController controller = mouse.GetComponent<MouseController>();
if (controller.caught) {
miceCaught++;
mice.Remove(mouse);
Destroy(mouse);
}
}
// Check if the game is over
if (miceCaught >= miceToWin) {
Debug.Log("Mice win!");
EndGame();
} else if (gameTimer <= 0f) {
Debug.Log("Cat wins!");
EndGame();
}
}
}
void EndGame () {
// Destroy all remaining mice
foreach (GameObject mouse in mice) {
Destroy(mouse);
}
mice.Clear();
// Destroy the cat
Destroy(cat);
// Restart the game
Start();
}
}
```
这个代码中,我们首先在 Start() 函数中生成一个猫和多个老鼠。然后,在 Update() 函数中,我们不断检查老鼠是否被猫抓住,如果有老鼠被抓住了,我们就将其从老鼠列表中删除,并将抓住的老鼠数量加1。如果猫抓住了足够数量的老鼠,老鼠赢得了游戏,否则猫赢得了游戏。
当游戏结束时,我们调用 EndGame() 函数来清理游戏场景,并重新开始游戏。
阅读全文
相关推荐
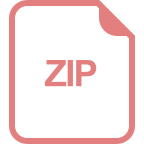
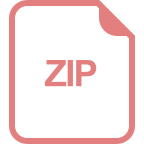
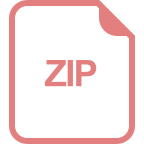
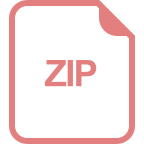
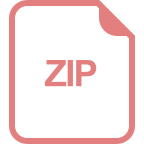
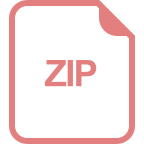
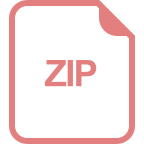
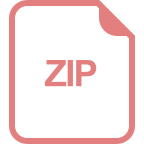
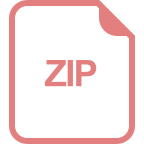
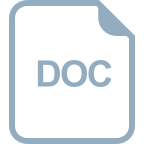

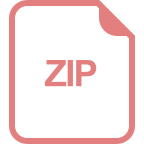
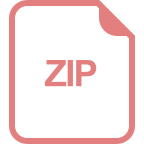