tamura纹理特征提取python
时间: 2023-11-23 17:07:58 浏览: 65
Tamura纹理特征提取是一种计算机视觉技术,用于描述图像的纹理特征。它可以用于图像分类、图像检索和图像分割等应用中。下面是使用Python实现Tamura纹理特征提取的示例代码:
```python
import cv2
import numpy as np
def tamura_features(image):
# Convert image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Compute Tamura features
f1 = tamura_coarseness(gray)
f2 = tamura_contrast(gray)
f3 = tamura_directionality(gray)
# Return feature vector
return np.array([f1, f2, f3])
def tamura_coarseness(image):
# Calculate the coarseness of the image
rows, cols = image.shape
coarseness = 0
for k in range(1, 4):
N = 2 ** (k + 1)
M = rows // N
L = cols // N
for i in range(0, M):
for j in range(0, L):
x1, y1 = i * N, j * N
x2, y2 = x1 + N, y1 + N
block = image[x1:x2, y1:y2]
var = np.var(block)
coarseness += var
coarseness /= 3 * M * L
return coarseness
def tamura_contrast(image):
# Calculate the contrast of the image
contrast = np.sum(np.abs(image.astype(np.float32) - np.mean(image))) / (image.shape[0] * image.shape[1])
return contrast
def tamura_directionality(image):
# Calculate the directionality of the image
rows, cols = image.shape
theta = np.pi / 4
dx = np.array([[1, 0], [1, 0], [1, 0], [0, 1], [0, 1], [-1, 0], [-1, 0], [-1, 0], [0, -1], [0, -1], [1, 1], [1, -1], [-1, 1], [-1, -1], [0, 0]], dtype=np.int32)
dy = np.array([[1, 0], [0, 1], [-1, 0], [1, 1], [0, 0], [-1, -1], [0, -1], [1, -1], [-1, 1], [0, 0], [0, 1], [-1, 0], [0, -1], [1, 0], [0, 0]], dtype=np.int32)
max_sum = 0
for k in range(0, 15):
sum = 0
for i in range(0, rows):
for j in range(0, cols):
if i + dx[k][0] >= 0 and i + dx[k][0] < rows and j + dx[k][1] >= 0 and j + dx[k][1] < cols and i + dy[k][0] >= 0 and i + dy[k][0] < rows and j + dy[k][1] >= 0 and j + dy[k][1] < cols:
sum += (image[i + dx[k][0], j + dx[k][1]] - image[i + dy[k][0], j + dy[k][1]]) ** 2
if sum > max_sum:
max_sum = sum
directionality = max_sum / (rows * cols)
return directionality
```
在这个示例中,`tamura_features`函数接受一个图像作为输入,并返回一个包含三个Tamura纹理特征的向量。这三个特征是:
- 粗糙度(coarseness):计算图像中每个局部区域的方差,并取其平均值。
- 对比度(contrast):计算图像中每个像素与图像均值之差的绝对值之和,并除以像素总数。
- 方向性(directionality):用15个不同的方向计算图像的局部方差,并取其中的最大值。
可以使用以下代码将这些特征应用于一张图像:
```python
image = cv2.imread('image.png')
features = tamura_features(image)
print(features)
```
这将输出一个包含三个特征值的向量,例如 `[0.123, 0.456, 0.789]`。
相关推荐











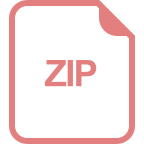