springboot整合druid数据源
时间: 2023-05-31 19:19:00 浏览: 207
### 回答1:
Spring Boot可以很方便地整合Druid数据源,只需要在pom.xml中添加Druid和JDBC依赖,然后在application.properties中配置Druid数据源即可。
具体步骤如下:
1. 在pom.xml中添加Druid和JDBC依赖:
```
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.1.10</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
```
2. 在application.properties中配置Druid数据源:
```
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&useSSL=false
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
# Druid配置
spring.datasource.type=com.alibaba.druid.pool.DruidDataSource
spring.datasource.druid.initial-size=5
spring.datasource.druid.min-idle=5
spring.datasource.druid.max-active=20
spring.datasource.druid.test-on-borrow=true
spring.datasource.druid.test-on-return=false
spring.datasource.druid.test-while-idle=true
spring.datasource.druid.time-between-eviction-runs-millis=60000
spring.datasource.druid.validation-query=SELECT 1 FROM DUAL
spring.datasource.druid.filters=stat,wall,log4j
spring.datasource.druid.max-wait=60000
spring.datasource.druid.pool-prepared-statements=true
spring.datasource.druid.max-pool-prepared-statement-per-connection-size=20
spring.datasource.druid.use-global-data-source-stat=true
```
3. 在代码中使用Druid数据源:
```
@Autowired
private DataSource dataSource;
```
以上就是整合Druid数据源的步骤,希望对你有所帮助。
### 回答2:
SpringBoot是现在使用最广泛的Java框架之一,它提供了很多方便开发的功能和快捷的开发方式,其中整合Druid数据源就是其中之一。
首先需要在pom.xml文件中引入druid和jdbc相关的依赖,例如:
```
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.10</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
```
接着,在application.properties文件中配置druid的数据源,例如:
```
# 数据源配置
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driverClassName=com.mysql.jdbc.Driver
spring.datasource.type=com.alibaba.druid.pool.DruidDataSource
spring.datasource.filters=stat,wall,log4j
spring.datasource.maxActive=20
spring.datasource.initialSize=1
spring.datasource.minIdle=3
spring.datasource.maxWait=60000
spring.datasource.timeBetweenEvictionRunsMillis=60000
spring.datasource.minEvictableIdleTimeMillis=300000
spring.datasource.validationQuery=SELECT 1 FROM DUAL
spring.datasource.poolPreparedStatements=true
spring.datasource.maxOpenPreparedStatements=50
```
其中,spring.datasource.url是数据库连接字符串,spring.datasource.username和spring.datasource.password是数据库的用户名和密码,spring.datasource.driverClassName是数据库驱动的类名。其他参数是Druid连接池的相关配置,比如最大并发连接数、初始连接数、最小空闲连接数等。
然后,通过在@SpringBootApplication注解中加上@EnableTransactionManagement和@MapperScan注解来开启事务和扫描Mapper,例如:
```
@SpringBootApplication
@EnableTransactionManagement
@MapperScan(basePackages = "com.example.demo.dao")
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
最后,在需要使用数据源的地方注入DataSource,并使用JdbcTemplate来操作数据库,例如:
```
@Service
public class UserServiceImpl implements UserService {
@Autowired
private DataSource dataSource;
private JdbcTemplate jdbcTemplate;
@PostConstruct
public void init() {
jdbcTemplate = new JdbcTemplate(dataSource);
}
@Override
public User getUserById(int id) {
String sql = "SELECT * FROM user WHERE id=?";
return jdbcTemplate.queryForObject(sql, new Object[]{id}, new BeanPropertyRowMapper<>(User.class));
}
@Override
public void saveUser(User user) {
String sql = "INSERT INTO user(name, age, gender) VALUES(?, ?, ?)";
jdbcTemplate.update(sql, new Object[]{user.getName(), user.getAge(), user.getGender()});
}
// 其他方法省略...
}
```
通过以上配置和使用,就能在SpringBoot项目中成功整合Druid数据源并操作数据库。
### 回答3:
Spring Boot 是一个快速构建 Spring 应用程序的框架,它内置了对多种数据源的支持,其中包括 Druid 数据源。Druid 是阿里巴巴开源的一款数据库连接池和 SQL 监控工具,它可以大大提高应用程序性能和数据库安全性。在本文中,我们将学习如何使用 Spring Boot 整合 Druid 数据源。
1. 引入依赖
在 pom.xml 中添加以下依赖:
```xml
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>${druid.version}</version>
</dependency>
```
2. 配置数据源
在 application.properties 或 application.yml 中配置 Druid 数据源:
```yaml
spring.datasource.url=jdbc:mysql://localhost:3306/test
spring.datasource.username=root
spring.datasource.password=root
# 连接池配置
spring.datasource.druid.initial-size=5
spring.datasource.druid.min-idle=5
spring.datasource.druid.max-active=20
spring.datasource.druid.max-wait=60000
spring.datasource.druid.time-between-eviction-runs-millis=60000
spring.datasource.druid.min-evictable-idle-time-millis=300000
spring.datasource.druid.validation-query=SELECT 1 FROM DUAL
spring.datasource.druid.test-while-idle=true
spring.datasource.druid.test-on-borrow=false
spring.datasource.druid.test-on-return=false
# 连接属性配置
spring.datasource.druid.filters=stat,wall
spring.datasource.druid.connection-properties=druid.stat.mergeSql=true;druid.stat.slowSqlMillis=5000
```
3. 配置 Druid 监控
可以通过以下配置开启 Druid 监控:
```yaml
# 监控统计
spring.datasource.druid.stat-view-servlet.enabled=true
spring.datasource.druid.stat-view-servlet.url-pattern=/druid/*
# 登录账号密码配置
spring.datasource.druid.stat-view-servlet.login-username=admin
spring.datasource.druid.stat-view-servlet.login-password=admin
# 过滤器配置
spring.datasource.druid.web-stat-filter.enabled=true
spring.datasource.druid.web-stat-filter.url-pattern=/*
spring.datasource.druid.web-stat-filter.exclusions=*.js,*.gif,*.jpg,*.png,*.css,*.ico,/druid/*
```
4. 使用数据源
现在我们可以通过注入 DataSource 对象来使用 Druid 数据源,例如:
```java
@RestController
public class TestController {
@Autowired
private DataSource dataSource;
// ...
}
```
以上就是使用 Spring Boot 整合 Druid 数据源的步骤。通过使用 Druid 数据源可以提高应用程序的性能和数据库安全性,而 Spring Boot 可以简化整个开发过程,让开发者更加专注于业务逻辑的实现。
阅读全文
相关推荐
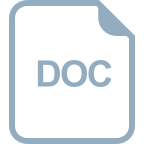
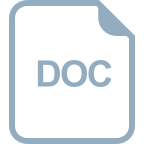
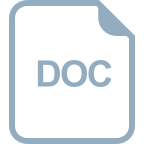
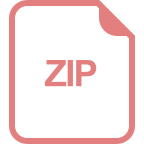
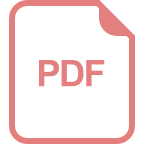
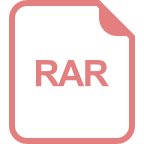
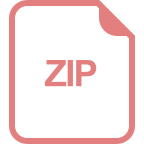
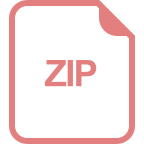
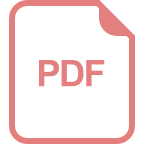
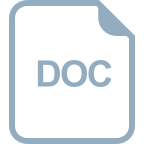



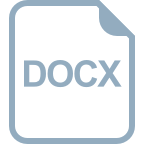
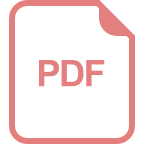
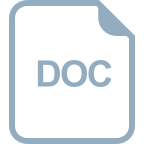


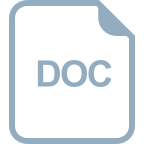