SQL使用游标编写,客户购买商品查询。 输入: 客户编号。 输出: 客户编号:128989 客户名称:丽丽 注册日期:2014-01-09 销售单号 产品编号 产品 单价 数量 总价 日期 -------------------------------------------------------------------------------------------------- 10909 p2323 帽子 12 1 12 2014-01-10 10909 x2933 皮鞋 100 2 200 2014-01-10 10919 m2333 手套 20 4 40 2014-08-09 ………………………. 商品销售情况统计。 输入:起始日期,截至日期 输出: 商品销售信息表 从 2014-01-01 到 2014-10-31 商品编号 商品名称 数量 合计金额 P09023 手表 120 120000 P09233 皮鞋 500 78000 .。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。 合计:7899999 元
时间: 2023-10-05 08:09:59 浏览: 91
以下是使用游标编写的客户购买商品查询和商品销售情况统计的 SQL 代码:
客户购买商品查询:
```
DECLARE @customer_id INT = 128989;
DECLARE @customer_name VARCHAR(50);
DECLARE @register_date DATE;
DECLARE @sales_order_number INT;
DECLARE @product_number VARCHAR(50);
DECLARE @product_name VARCHAR(50);
DECLARE @product_price DECIMAL(10, 2);
DECLARE @quantity INT;
DECLARE @total_price DECIMAL(10, 2);
DECLARE @sale_date DATE;
DECLARE sales_cursor CURSOR FOR
SELECT c.customer_name, c.register_date, s.sales_order_number, s.product_number, p.product_name, p.product_price, s.quantity, s.total_price, s.sale_date
FROM customers c
JOIN sales s ON c.customer_id = s.customer_id
JOIN products p ON s.product_number = p.product_number
WHERE c.customer_id = @customer_id;
OPEN sales_cursor;
FETCH NEXT FROM sales_cursor INTO
@customer_name, @register_date, @sales_order_number, @product_number, @product_name, @product_price, @quantity, @total_price, @sale_date;
PRINT '客户编号: ' + CAST(@customer_id AS VARCHAR(10));
PRINT '客户名称: ' + @customer_name;
PRINT '注册日期: ' + CONVERT(VARCHAR(10), @register_date, 120);
PRINT '销售单号' + ' ' + '产品编号' + ' ' + '产品' + ' ' + '单价' + ' ' + '数量' + ' ' + '总价' + ' ' + '日期';
PRINT '--------------------------------------------------------------------------------------------------';
WHILE @@FETCH_STATUS = 0
BEGIN
PRINT CAST(@sales_order_number AS VARCHAR(10)) + ' ' + @product_number + ' ' + @product_name + ' ' + CAST(@product_price AS VARCHAR(10)) + ' ' + CAST(@quantity AS VARCHAR(10)) + ' ' + CAST(@total_price AS VARCHAR(10)) + ' ' + CONVERT(VARCHAR(10), @sale_date, 120);
FETCH NEXT FROM sales_cursor INTO
@customer_name, @register_date, @sales_order_number, @product_number, @product_name, @product_price, @quantity, @total_price, @sale_date;
END;
CLOSE sales_cursor;
DEALLOCATE sales_cursor;
```
商品销售情况统计:
```
DECLARE @start_date DATE = '2014-01-01';
DECLARE @end_date DATE = '2014-10-31';
DECLARE @product_number VARCHAR(50);
DECLARE @product_name VARCHAR(50);
DECLARE @quantity INT;
DECLARE @total_price DECIMAL(10, 2);
DECLARE @grand_total DECIMAL(10, 2) = 0;
DECLARE product_sales_cursor CURSOR FOR
SELECT s.product_number, p.product_name, SUM(s.quantity), SUM(s.total_price)
FROM sales s
JOIN products p ON s.product_number = p.product_number
WHERE s.sale_date BETWEEN @start_date AND @end_date
GROUP BY s.product_number, p.product_name
ORDER BY s.product_number;
PRINT '商品销售信息表';
PRINT '从 ' + CONVERT(VARCHAR(10), @start_date, 120) + ' 到 ' + CONVERT(VARCHAR(10), @end_date, 120);
PRINT '商品编号' + ' ' + '商品名称' + ' ' + '数量' + ' ' + '合计金额';
PRINT '-----------------------------------------';
OPEN product_sales_cursor;
FETCH NEXT FROM product_sales_cursor INTO
@product_number, @product_name, @quantity, @total_price;
WHILE @@FETCH_STATUS = 0
BEGIN
PRINT @product_number + ' ' + @product_name + ' ' + CAST(@quantity AS VARCHAR(10)) + ' ' + CAST(@total_price AS VARCHAR(10));
SET @grand_total = @grand_total + @total_price;
FETCH NEXT FROM product_sales_cursor INTO
@product_number, @product_name, @quantity, @total_price;
END;
PRINT '合计: ' + CAST(@grand_total AS VARCHAR(10)) + ' 元';
CLOSE product_sales_cursor;
DEALLOCATE product_sales_cursor;
```
请注意,游标不是 SQL 中的最佳实践,因为它们在处理大量数据时可能会导致性能问题。如果可能的话,最好使用其他 SQL 技术来处理数据。
阅读全文
相关推荐



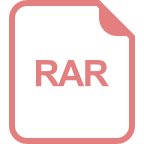


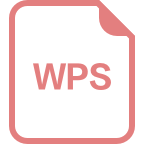
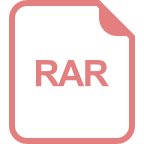

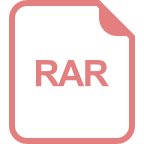




