写一个在unity中解析ply格式模型的顶点坐标、顶点索引、颜色信息等信息的脚本
时间: 2024-03-08 17:47:29 浏览: 25
下面是一个简单的解析ply格式模型的脚本,可以获取顶点坐标、顶点索引和颜色信息。
```csharp
using UnityEngine;
using System.IO;
using System.Collections.Generic;
public class PlyLoader : MonoBehaviour
{
public string filePath; // ply文件路径
private List<Vector3> vertices = new List<Vector3>(); // 顶点列表
private List<int> indices = new List<int>(); // 索引列表
private List<Color32> colors = new List<Color32>(); // 颜色列表
private void Start()
{
LoadPlyFile();
}
private void LoadPlyFile()
{
if (File.Exists(filePath))
{
StreamReader reader = new StreamReader(filePath);
// 读取文件头
string line = reader.ReadLine();
while (line != null && !line.Contains("end_header"))
{
if (line.Contains("element vertex"))
{
int vertexCount = int.Parse(line.Split(' ')[2]);
}
line = reader.ReadLine();
}
// 读取顶点数据
for (int i = 0; i < vertexCount; i++)
{
string[] splitLine = reader.ReadLine().Split(' ');
// 读取顶点坐标
float x = float.Parse(splitLine[0]);
float y = float.Parse(splitLine[1]);
float z = float.Parse(splitLine[2]);
vertices.Add(new Vector3(x, y, z));
// 读取颜色信息
byte r = byte.Parse(splitLine[3]);
byte g = byte.Parse(splitLine[4]);
byte b = byte.Parse(splitLine[5]);
colors.Add(new Color32(r, g, b, 255));
}
// 读取面数据
while (line != null)
{
string[] splitLine = line.Split(' ');
if (splitLine[0] == "3")
{
int i0 = int.Parse(splitLine[1]);
int i1 = int.Parse(splitLine[2]);
int i2 = int.Parse(splitLine[3]);
indices.Add(i0);
indices.Add(i1);
indices.Add(i2);
}
line = reader.ReadLine();
}
reader.Close();
// 创建网格并设置数据
Mesh mesh = new Mesh();
mesh.vertices = vertices.ToArray();
mesh.triangles = indices.ToArray();
mesh.colors32 = colors.ToArray();
// 设置网格并添加到场景中
GetComponent<MeshFilter>().mesh = mesh;
GetComponent<MeshRenderer>().material = new Material(Shader.Find("Standard"));
}
else
{
Debug.LogError("File does not exist.");
}
}
}
```
请注意,这只是一个简单的示例脚本,仅支持一些基本的ply格式,可能无法处理复杂的ply文件。如果需要更复杂的解析,可能需要编写更高级的代码。
相关推荐
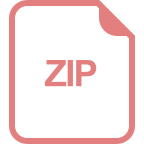














