请使用深度学习中的卷积神经网络(CNN)帮我构建一个数字识别器,可以将手写数字识别出来。
时间: 2024-03-17 18:40:10 浏览: 15
好的,我可以帮您实现一个基于CNN的手写数字识别器。我们可以使用Python编程语言和深度学习框架TensorFlow来构建这个模型。
首先,我们需要导入必要的库:
```python
import tensorflow as tf
from tensorflow.keras import datasets, layers, models
import matplotlib.pyplot as plt
```
然后,我们可以加载MNIST数据集并将其划分为训练集和测试集:
```python
(train_images, train_labels), (test_images, test_labels) = datasets.mnist.load_data()
```
接下来,我们可以对图像数据进行预处理,将像素值缩放到0到1之间,并将图像数据从3D张量转换为4D张量:
```python
train_images = train_images / 255.0
test_images = test_images / 255.0
train_images = train_images.reshape((train_images.shape[0], 28, 28, 1))
test_images = test_images.reshape((test_images.shape[0], 28, 28, 1))
```
然后,我们可以构建CNN模型。这里我们使用两个卷积层,每个卷积层后面跟着一个池化层,以及一个全连接层和一个输出层:
```python
model = models.Sequential()
model.add(layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)))
model.add(layers.MaxPooling2D((2, 2)))
model.add(layers.Conv2D(64, (3, 3), activation='relu'))
model.add(layers.MaxPooling2D((2, 2)))
model.add(layers.Flatten())
model.add(layers.Dense(64, activation='relu'))
model.add(layers.Dense(10))
```
最后,我们可以编译模型并训练它:
```python
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
history = model.fit(train_images, train_labels, epochs=10,
validation_data=(test_images, test_labels))
```
训练完成后,我们可以使用测试集评估模型的性能:
```python
test_loss, test_acc = model.evaluate(test_images, test_labels, verbose=2)
print("Test accuracy:", test_acc)
```
最后,我们可以使用模型来预测手写数字图像:
```python
import numpy as np
# Load a sample image and preprocess it
image = plt.imread("example.png")
image = np.mean(image, axis=2)
image = np.expand_dims(image, axis=0)
image = np.expand_dims(image, axis=-1)
image = image / 255.0
# Make a prediction
prediction = model.predict(image)
digit = np.argmax(prediction)
print("The predicted digit is:", digit)
```
这样,我们就成功地构建了一个基于CNN的手写数字识别器。
相关推荐
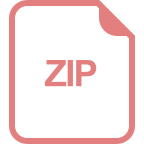
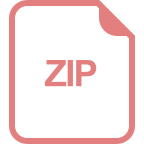
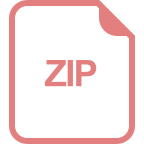














