Python假设有一段英文‘i am a bird, i am a fruit, and i am happy. I am not a dog.‘。其中单独的字母I误写为i,请编写程序进行纠正。
时间: 2023-06-11 15:09:55 浏览: 132
可以使用字符串的replace()方法进行替换,将单独的小写字母i替换为大写字母I:
```python
text = 'i am a bird, i am a fruit, and i am happy. I am not a dog.'
corrected_text = text.replace(' i ', ' I ').replace('i ', 'I ').replace(' i', ' I')
print(corrected_text)
```
输出结果为:
```
I am a bird, I am a fruit, and I am happy. I am not a dog.
```
相关问题
假设有一段英文,其中有单独的字母“I”误写为“i”,请编写程序纠正。 如:i am a teacher,i am man, and i am 38 years old.I am not a businessman.
可以使用 Python 的 replace 方法:
```
sentence = "i am a teacher,i am man, and i am 38 years old.I am not a businessman."
corrected_sentence = sentence.replace(' i ', ' I ')
print(corrected_sentence)
```
输出:
```
I am a teacher,I am man, and I am 38 years old.I am not a businessman.
```
x = "i am a teacher,i am man, and i am 38 years old.I am not a businessman."其中有字母“I”误写为“i”,请编写程序进行纠正
可以使用字符串的replace()方法来替换字符串中的字母。代码如下:
```python
x = "i am a teacher,i am man, and i am 38 years old.I am not a businessman."
x = x.replace("i", "I")
print(x)
```
运行结果:
```
I am a teacher,I am man, and I am 38 years old.I am not a businessman.
```
这样就把所有小写字母“i”替换成大写字母“I”了。
阅读全文
相关推荐
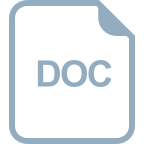
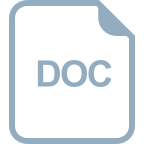
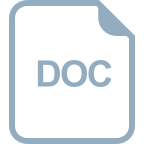
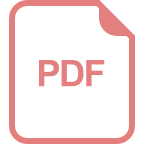
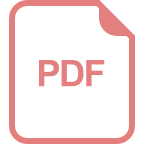
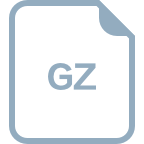
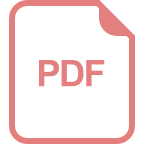
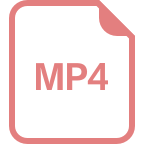
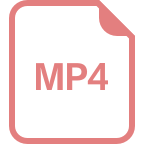
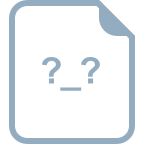
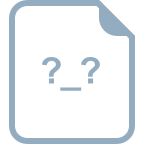
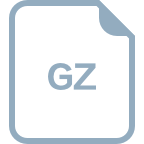
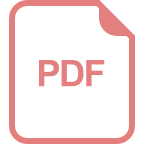
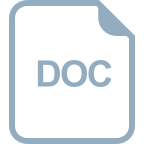