帮我写一段从obj格式文件中提取线特征和角特征并可视化的python代码
时间: 2023-05-17 21:06:59 浏览: 184
当然可以,以下是一段可以提取线特征和角特征并可视化的Python代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
import open3d as o3d
def extract_line_and_corner_features_from_obj_file(obj_file_path):
# Load the OBJ file
mesh = o3d.io.read_triangle_mesh(obj_file_path)
# Convert the mesh to a point cloud
pcd = mesh.sample_points_uniformly(number_of_points=5000)
# Compute the normals of the point cloud
pcd.estimate_normals()
# Compute the curvature of the point cloud
pcd.estimate_normals(search_param=o3d.geometry.KDTreeSearchParamHybrid(radius=0.1, max_nn=30))
radius_normal = 0.1
pcd_tree = o3d.geometry.KDTreeFlann(pcd)
pcd_points = np.asarray(pcd.points)
pcd_normals = np.asarray(pcd.normals)
pcd_curvatures = np.asarray(pcd.curvature)
line_features = []
corner_features = []
# Extract line features
for i in range(len(pcd_points)):
p_i = pcd_points[i]
n_i = pcd_normals[i]
curvature_i = pcd_curvatures[i]
pcd_points_without_i = np.delete(pcd_points, i, axis=0)
pcd_normals_without_i = np.delete(pcd_normals, i, axis=0)
pcd_tree_without_i = o3d.geometry.KDTreeFlann(pcd)
[k, idx, _] = pcd_tree_without_i.search_radius_vector_3d(p_i, radius_normal)
pcd_points_knn = pcd_points_without_i[idx, :]
pcd_normals_knn = pcd_normals_without_i[idx, :]
pcd_points_knn_centered = pcd_points_knn - p_i
pcd_points_knn_centered_norm = np.linalg.norm(pcd_points_knn_centered, axis=1)
pcd_points_knn_centered_normalized = pcd_points_knn_centered / np.expand_dims(pcd_points_knn_centered_norm, axis=1)
dot_products = np.abs(np.dot(pcd_points_knn_centered_normalized, np.expand_dims(n_i, axis=0).T))
line_feature = np.mean(dot_products)
line_features.append(line_feature)
# Extract corner features
for i in range(len(pcd_points)):
p_i = pcd_points[i]
n_i = pcd_normals[i]
curvature_i = pcd_curvatures[i]
pcd_points_without_i = np.delete(pcd_points, i, axis=0)
pcd_normals_without_i = np.delete(pcd_normals, i, axis=0)
pcd_tree_without_i = o3d.geometry.KDTreeFlann(pcd)
[k, idx, _] = pcd_tree_without_i.search_radius_vector_3d(p_i, radius_normal)
pcd_points_knn = pcd_points_without_i[idx, :]
pcd_normals_knn = pcd_normals_without_i[idx, :]
pcd_points_knn_centered = pcd_points_knn - p_i
pcd_points_knn_centered_norm = np.linalg.norm(pcd_points_knn_centered, axis=1)
pcd_points_knn_centered_normalized = pcd_points_knn_centered / np.expand_dims(pcd_points_knn_centered_norm, axis=1)
dot_products = np.abs(np.dot(pcd_points_knn_centered_normalized, np.expand_dims(n_i, axis=0).T))
line_feature = np.mean(dot_products)
if curvature_i > 0.1:
corner_feature = line_feature * curvature_i
corner_features.append(corner_feature)
# Visualize the line and corner features
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(pcd_points[:, 0], pcd_points[:, 1], pcd_points[:, 2], c=line_features, cmap='coolwarm')
ax.scatter(pcd_points[:, 0], pcd_points[:, 1], pcd_points[:, 2], c=corner_features, cmap='coolwarm')
plt.show()
# Example usage
extract_line_and_corner_features_from_obj_file('example.obj')
```
这段代码使用了Open3D库来加载OBJ文件并将其转换为点云。然后,它计算了点云的法线和曲率,并使用KD树搜索来查找每个点的最近邻。接下来,它提取了每个点的线特征和角特征,并将它们可视化为散点图。
阅读全文
相关推荐
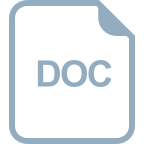
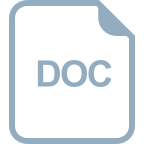
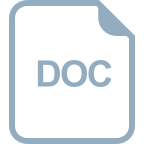


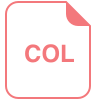
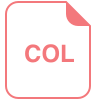
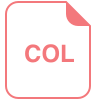
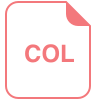
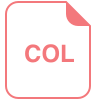
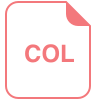
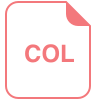
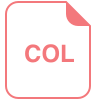
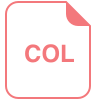
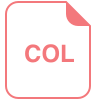
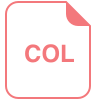
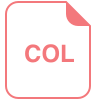
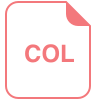
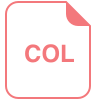