python 用counter
时间: 2024-05-02 11:03:17 浏览: 93
Counter 是 Python 中的一个内置类,用于计算可迭代对象中每个元素出现的次数。它可以用于任何可迭代对象,例如字符串、列表、元组等。
下面是一个示例,使用 Counter 统计一段文本中每个单词出现的次数:
```python
from collections import Counter
text = "Python is a high-level programming language. It is used for web development, data analysis, artificial intelligence, and more."
# 将文本分割成单词,并统计每个单词出现的次数
word_counts = Counter(text.split())
# 输出结果
print(word_counts)
```
输出结果:
```
Counter({'is': 2, 'Python': 1, 'a': 1, 'high-level': 1, 'programming': 1, 'language.': 1, 'It': 1, 'used': 1, 'for': 1, 'web': 1, 'development,': 1, 'data': 1, 'analysis,': 1, 'artificial': 1, 'intelligence,': 1, 'and': 1, 'more.': 1})
```
可以看到,Counter 返回了一个字典,其中每个单词是一个键,对应的值是它在文本中出现的次数。
除了使用 split() 方法将文本分割成单词,还可以使用正则表达式等方式进行分割。例如,下面的代码使用 re 模块将文本分割成单词和标点符号:
```python
import re
from collections import Counter
text = "Python is a high-level programming language. It is used for web development, data analysis, artificial intelligence, and more."
# 将文本分割成单词和标点符号,并统计每个单词出现的次数
word_counts = Counter(re.findall('\w+|[^\w\s]+', text))
# 输出结果
print(word_counts)
```
输出结果:
```
Counter({'is': 2, 'Python': 1, 'a': 1, 'high': 1, '-': 1, 'level': 1, 'programming': 1, 'language': 1, '.': 1, 'It': 1, 'used': 1, 'for': 1, 'web': 1, 'development': 1, ',': 1, 'data': 1, 'analysis': 1, 'artificial': 1, 'intelligence': 1, 'and': 1, 'more': 1, '.': 1})
```
可以看到,使用正则表达式进行分割后,结果中包含了标点符号,并且点号(.)出现了两次。
阅读全文
相关推荐
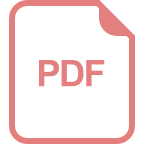
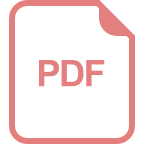
















