c++重载运算符后为什么还是无法访问私有变量
时间: 2023-07-05 19:03:19 浏览: 76
在 C++ 中,类的私有成员变量只能在类的成员函数中被访问。即使你在重载运算符时使用了类的成员函数,如果该成员函数没有被声明为类的友元函数,那么它也无法访问该类的私有成员变量。因此,如果你想在重载运算符中访问类的私有成员变量,你可以考虑将该运算符重载函数声明为该类的友元函数。或者,你可以添加公共成员函数来访问私有成员变量,并在运算符重载函数中调用该公共成员函数。
相关问题
c++重载运算符复数
在 C++ 中,可以通过重载运算符来定义复数类型的加法、减法、乘法和除法等运算。以下是一个示例代码:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real, imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(const Complex& c) const {
return Complex(real + c.real, imag + c.imag);
}
Complex operator-(const Complex& c) const {
return Complex(real - c.real, imag - c.imag);
}
Complex operator*(const Complex& c) const {
return Complex(real * c.real - imag * c.imag, real * c.imag + imag * c.real);
}
Complex operator/(const Complex& c) const {
double denominator = c.real * c.real + c.imag * c.imag;
return Complex((real * c.real + imag * c.imag) / denominator, (imag * c.real - real * c.imag) / denominator);
}
friend ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
};
int main() {
Complex c1(1, 2), c2(2, 3);
cout << "c1=" << c1 << endl;
cout << "c2=" << c2 << endl;
cout << "c1+c2=" << c1 + c2 << endl;
cout << "c1-c2=" << c1 - c2 << endl;
cout << "c1*c2=" << c1 * c2 << endl;
cout << "c1/c2=" << c1 / c2 << endl;
return 0;
}
```
在上述代码中,我们定义了一个名为 Complex 的类,用于表示复数。该类包含了两个私有成员变量 real 和 imag,分别表示实部和虚部。我们重载了加法运算符 operator+、减法运算符 operator-、乘法运算符 operator* 和除法运算符 operator/,并且实现了一个友元函数 operator<<,用于输出复数类型。
在主函数中,我们创建了两个复数对象 c1 和 c2,然后进行加、减、乘、除等运算,并输出结果。
c++重载运算符技巧
C++中的运算符重载是一种特殊的多态性,它允许我们重新定义已有的运算符,以便用于用户自定义的数据类型。以下是一些C++重载运算符的技巧:
1. 运算符重载函数必须是类的成员函数或全局函数,但不能是普通的成员函数。
2. 重载运算符函数的名称必须是operator后面跟着要重载的运算符符号,例如+、-、*、/等。
3. 重载运算符函数可以有任意数量的参数,但必须至少有一个参数是类类型或枚举类型。
4. 重载运算符函数可以返回任何类型的值,但通常返回一个新的类对象。
5. 重载运算符函数可以是const函数,这意味着它不会修改类的成员变量。
6. 重载运算符函数可以是友元函数,这意味着它可以访问类的私有成员变量。
以下是一个C++重载运算符的例子:
```cpp
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
Complex operator+(const Complex& c) const {
return Complex(real + c.real, imag + c.imag);
}
friend ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
private:
double real;
double imag;
};
int main() {
Complex c1(1.0, 2.0);
Complex c2(3.0, 4.0);
Complex c3 = c1 + c2;
cout << c3 << endl; // 输出:4+6i
return 0;
}
```
阅读全文
相关推荐
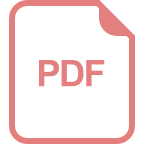
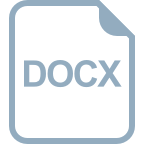
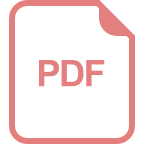
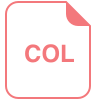


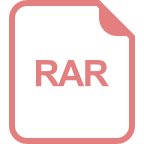
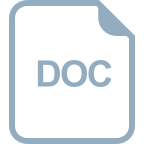
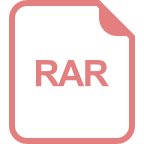
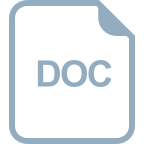
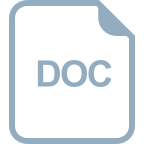




