c#栈,如何遍历栈中的元素而不出栈
时间: 2024-09-26 22:11:06 浏览: 44
在 C# 中,栈是一种只能在一端进行插入和删除操作的数据结构,通常遵循 Last In First Out (LIFO) 的原则。如果你想遍历栈中的元素而不改变栈的内容(即不出栈),你可以通过迭代的方式来完成。这里是一个简单的例子:
```csharp
Stack<int> stack = new Stack<int>(); // 创建一个整数栈
// 先将元素压入栈中
stack.Push(1);
stack.Push(2);
stack.Push(3);
// 使用 Stack 的 GetEnumerator 或者 foreach 遍历元素
IEnumerator<int> iterator = stack.GetEnumerator(); // 获取枚举器
while (iterator.MoveNext()) {
int item = iterator.Current; // 获取当前栈顶元素并处理它,但不会出栈
Console.WriteLine(item);
}
// 输出:3 2 1
```
在这个示例中,我们并没有用 `Pop()` 函数取出元素,而是通过 `GetEnumerator()` 获取了堆栈的迭代器,然后在循环中逐步访问每个元素。这种方式可以避免直接从栈中移除元素,保持栈内容不变。
相关问题
如何使用C#控制台实现一个基于栈数据结构的表达式括号匹配器?包括检查栈是否为空、执行入栈操作、出栈操作以及获取栈顶元素的功能,同时处理正确的括号配对逻辑。
在C#中,你可以创建一个`Stack<char>`来存储输入的字符,其中`char`是用于表示括号的数据类型。下面是一个基本的步骤说明:
1. 定义一个名为`BracketMatcher`的类,包含一个`Stack<char>`类型的实例变量`openBrackets`来保存打开的括号。
```csharp
using System;
using System.Collections.Generic;
public class BracketMatcher
{
private Stack<char> openBrackets = new Stack<char>();
}
```
2. 创建入栈(push)方法,当遇到开放括号('(', '[', '{')时,将其压入栈中。
```csharp
public void Push(char bracket)
{
if (IsOpening(bracket))
{
openBrackets.Push(bracket);
}
}
```
3. 出栈(pop)方法,当遍历到关闭括号时,检查栈顶元素是否与其匹配。如果是,则弹出;如果不是,表示未闭合的括号错误。
```csharp
private bool IsClosing(char closingBracket)
{
switch (closingBracket)
{
case ')':
return openBrackets.Peek() == '(';
case ']':
return openBrackets.Peek() == '[';
case '}':
return openBrackets.Peek() == '{';
default:
return false;
}
}
public void Pop()
{
if (!openBrackets.isEmpty())
{
char topBracket = openBrackets.Pop();
if (!IsClosing(topBracket))
{
// 括号不匹配,记录错误
Console.WriteLine($"Error: Mismatched brackets ({topBracket})");
}
}
else
{
throw new InvalidOperationException("Tried to pop from an empty stack");
}
}
```
4. 检查栈是否为空。可以使用`isEmpty()`方法,但在C#中需要自定义这个方法,因为`Stack<T>`类本身没有提供此功能。
```csharp
public bool IsEmpty()
{
return openBrackets.Count == 0;
}
```
5. 获取栈顶元素,同样如果栈为空则抛异常。
```csharp
public char Peek()
{
if (IsEmpty())
{
throw new InvalidOperationException("Cannot peek at an empty stack");
}
return openBrackets.Peek();
}
```
6. 最后,遍历整个输入字符串,调用上述方法进行匹配。如果有错误的括号组合,可以在遍历结束后输出所有找到的问题。
为了判断整个表达式是否有效,你可以将上述方法放入一个`MatchExpression`方法中,并返回一个布尔值表示是否所有括号都已正确匹配。
```csharp
public bool MatchExpression(string expression)
{
foreach (char c in expression)
{
if (char.IsDigit(c) || char.IsLetter(c) || c == '.')
{
// 对于数字、字母或点,直接忽略
}
else
{
Push(c);
}
}
while (!openBrackets.IsEmpty())
{
Pop();
}
return openBrackets.IsEmpty();
}
```
输出所有出栈的可能C#
在C#中,如果你想要模拟一个栈的数据结构,并输出所有出栈的可能顺序,你可以创建一个栈并在循环中不断弹出元素并打印出来。这里是一个简单的例子,假设我们有一个整数栈:
```csharp
using System;
using System.Collections.Generic;
public class StackExample
{
private List<int> stack = new List<int>();
// 入栈操作
public void Push(int value)
{
stack.Add(value);
}
// 出栈操作
public int Pop()
{
if (stack.Count == 0) throw new InvalidOperationException("Stack is empty");
return stack.RemoveAt(stack.Count - 1);
}
// 打印出栈的所有可能顺序
public void PrintAllPossibleOutpus()
{
for (int i = stack.Count - 1; i >= 0; i--)
{
Console.WriteLine($"Output #{i + 1}: {stack[i]}");
}
}
}
public class Program
{
public static void Main(string[] args)
{
var stackObj = new StackExample();
// 假设这里先入栈了几个数字
stackObj.Push(1);
stackObj.Push(2);
stackObj.Push(3);
stackObj.PrintAllPossibleOutpus();
}
}
```
在这个程序中,`Push`方法用于向栈中添加元素,`Pop`方法用于移除并返回栈顶元素。`PrintAllPossibleOutpus`方法会遍历栈,从最后一个元素开始逐个出栈并打印。
阅读全文
相关推荐






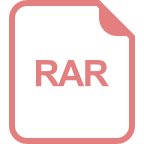









