优化下列代码 #include<iostream> #include<bits/stdc++.h> using namespace std; struct Student { string name; int scroe; }a[7]; bool compare (Student.scroe,Student.scroe) { return a.scroe>b.scroe; } int main() { int m; cin>>m; for(int i=0;i<m;i++) { cin>>a[i].name; } for(int i=0;i<m;i++) { cin>>a[i].scroe; } sort(a[0].scroe,a[m-1].score,compare); for(int i=0;i<m;i++) { cout<<a[i].name<<" "; } }
时间: 2023-07-31 22:09:42 浏览: 360
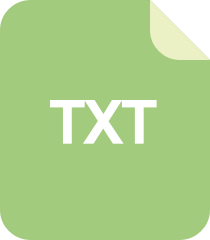
#include_iostream.h_

#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
struct Student {
string name;
int score;
};
bool compare(const Student& a, const Student& b) {
return a.score > b.score;
}
int main() {
int m;
cin >> m;
vector<Student> students(m);
for (int i = 0; i < m; i++) {
cin >> students[i].name;
}
for (int i = 0; i < m; i++) {
cin >> students[i].score;
}
sort(students.begin(), students.end(), compare);
for (int i = 0; i < m; i++) {
cout << students[i].name << " ";
}
return 0;
}
阅读全文
相关推荐
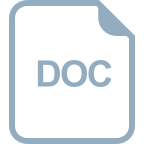
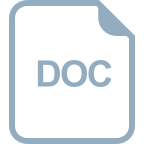
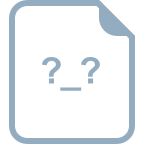


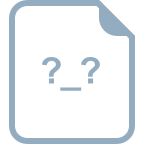
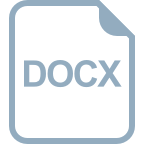
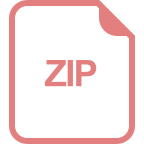
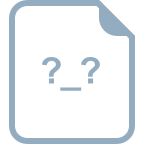
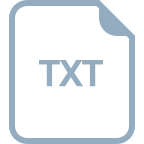
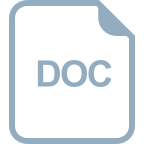
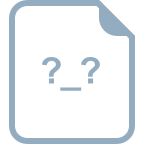
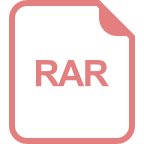
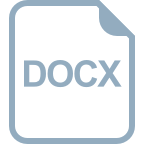
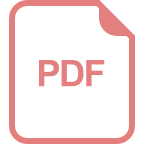
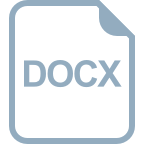
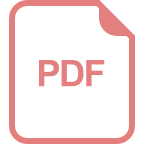