python random forests
时间: 2023-10-20 10:35:01 浏览: 120
Random forests are an ensemble learning method in machine learning that combine multiple decision trees to make more accurate predictions. In the case of Python, you can use the scikit-learn library to implement random forests.
Here's an example of how to use random forests in Python:
1. First, you need to install the scikit-learn library if you haven't already. You can do this by running the following command:
```
pip install scikit-learn
```
2. Once you have scikit-learn installed, you can import the necessary classes and functions:
```python
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
```
3. Next, you need to prepare your data. Random forests can be used for both classification and regression tasks. For classification, you'll need a set of labeled data, where each data point has a set of features and a corresponding class label.
4. Split your dataset into training and testing sets using the `train_test_split` function:
```python
X_train, X_test, y_train, y_test = train_test_split(features, labels, test_size=0.2)
```
5. Create an instance of the `RandomForestClassifier` class and fit it to your training data:
```python
clf = RandomForestClassifier()
clf.fit(X_train, y_train)
```
6. Once the model is trained, you can use it to make predictions on new data:
```python
y_pred = clf.predict(X_test)
```
7. Finally, evaluate the accuracy of your model by comparing the predicted labels with the actual labels:
```python
accuracy = accuracy_score(y_test, y_pred)
```
That's a basic overview of using random forests in Python with scikit-learn. Remember to adjust the parameters of the RandomForestClassifier class, such as the number of trees, to optimize the performance of your model.
阅读全文
相关推荐
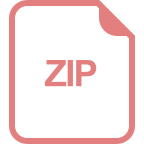
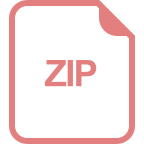
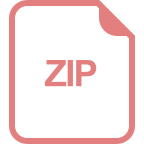
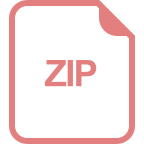



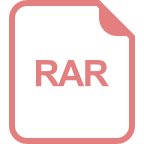
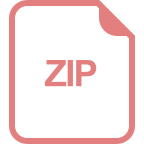
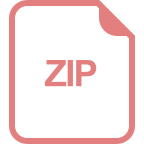
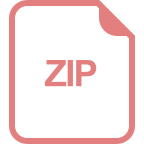



